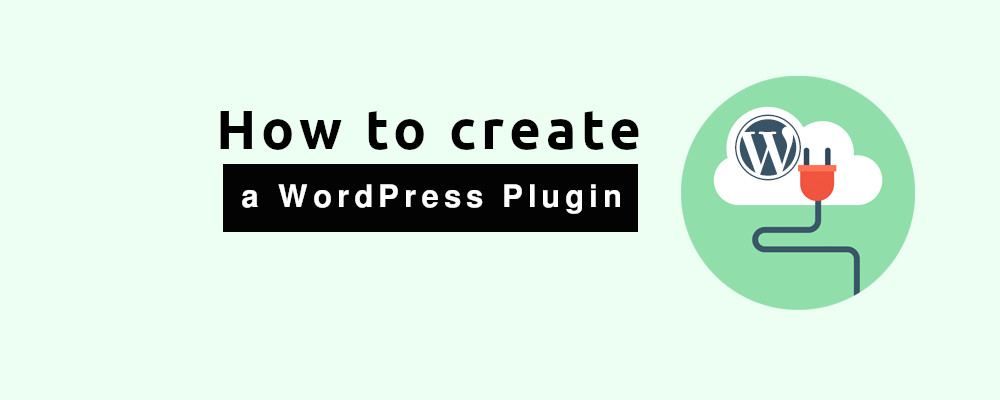
How to create a WordPress Plugin for beginners
WordPress is an incredibly versatile platform that allows users to extend its functionality through plugins. In this article, we will learn how to create a basic plugin from scratch, step by step, with code examples in both Spanish and English.
1: Setting up the Development Environment.
Before we begin, we need to have a development environment set up. Make sure you have a web server with WordPress installed and ready to use.
2: Basic Plugin Structure.
Let’s start by creating the basic structure of our plugin. Create a folder in the WordPress plugins directory, and inside it, create a main file with the plugin information.
// my-plugin/my-plugin.php <?php /* Plugin Name: My Plugin Description: A brief plugin description. Version: 1.0 Author: Your Name Author URI: https://blog.letsgodev.com License: GPL2 License URI: https://www.gnu.org/licenses/gpl-2.0.html Text Domain: wp-tutorial Domain Path: /languages */ // Plugin code goes here ?>
3: Adding Functionality.
Let’s add basic functionality to the plugin, for example, displaying a custom message in the admin bar.
// my-plugin/my-plugin.php <?php // ... (previous code) // Function to display the message in the admin bar function show_admin_message() { echo '<div class="notice notice-success is-dismissible"> <p>Hello, this is your custom message.</p> </div>'; } // Hook to call the function add_action('admin_notices', 'show_admin_message'); // ... (subsequent code) ?>
4: Plugin Activation and Deactivation.
Let’s add hooks to execute functions when the plugin is activated or deactivated.
// my-plugin/my-plugin.php <?php // ... (previous code) // Function to perform actions when the plugin is activated function activate_my_plugin() { // Activation actions here } // Function to perform actions when the plugin is deactivated function deactivate_my_plugin() { // Deactivation actions here } // Activation and deactivation hooks register_activation_hook(__FILE__, 'activate_my_plugin'); register_deactivation_hook(__FILE__, 'deactivate_my_plugin'); // ... (subsequent code) ?>
5: Hooks.
<?php function replace_wordpress_word( $content = '' ) { return str_replace( 'wordpress', 'WordPress', $content ); } add_filter( 'the_content', 'replace_wordpress_word' );
Conclusion.
This article provides a basic introduction on how to create a plugin for WordPress. You can expand and customize this foundation according to your specific needs. I hope you find this tutorial helpful!
Remember to adjust and customize the code according to the specific requirements of your plugin. Good luck with your development!