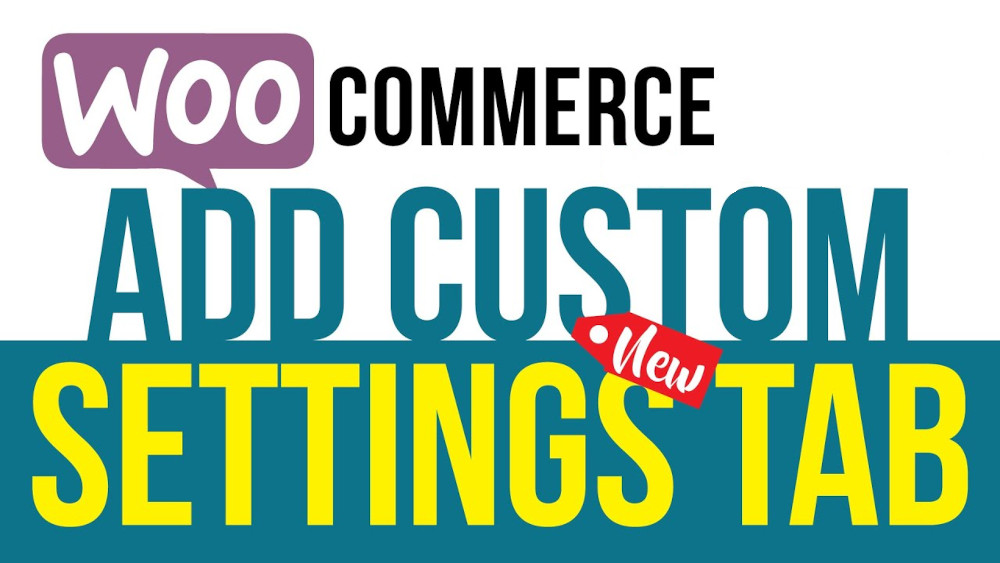
Adding a custom tab to the WooCommerce settings page
WooCommerce offers great flexibility for customizing its functionality. One aspect that can be customized is the settings page, where you can add custom tabs with your own settings. To do this, we will use the woocommerce_get_settings_pages
hook.
Create a basic tab
First, let’s add a basic tab to the WooCommerce settings page.
1) Add the function to register the custom settings page:
<?php add_filter('woocommerce_get_settings_pages', 'add_custom_settings_page'); function add_custom_settings_page($settings) { $settings[] = include 'class-wc-settings-custom.php'; return $settings; }
2) Create the class-wc-settings-custom.php
file:
This file will contain the class that defines our custom tab.
<?php if (!class_exists('WC_Settings_Page')) { return; } class WC_Settings_Custom extends WC_Settings_Page { public function __construct() { $this->id = 'custom_settings'; $this->label = __('Custom Settings', 'woocommerce'); add_filter('woocommerce_settings_tabs_array', array($this, 'add_settings_page'), 20); add_action('woocommerce_settings_' . $this->id, array($this, 'output')); add_action('woocommerce_settings_save_' . $this->id, array($this, 'save')); } public function get_sections() { $sections = array( '' => __('General', 'woocommerce'), 'advanced' => __('Advanced', 'woocommerce') ); return $sections; } public function get_settings($current_section = '') { if ($current_section == 'advanced') { return array( 'section_title' => array( 'name' => __('Advanced Section Title', 'woocommerce'), 'type' => 'title', 'desc' => '', 'id' => 'advanced_section_title' ), 'custom_text_field' => array( 'name' => __('Advanced Text Field', 'woocommerce'), 'type' => 'text', 'desc' => __('Enter some text', 'woocommerce'), 'id' => 'advanced_text_field' ), 'section_end' => array( 'type' => 'sectionend', 'id' => 'advanced_section_end' ), ); } else { return array( 'section_title' => array( 'name' => __('General Section Title', 'woocommerce'), 'type' => 'title', 'desc' => '', 'id' => 'general_section_title' ), 'custom_text_field' => array( 'name' => __('General Text Field', 'woocommerce'), 'type' => 'text', 'desc' => __('Enter some text', 'woocommerce'), 'id' => 'general_text_field' ), 'custom_number_field' => array( 'name' => __('General Number Field', 'woocommerce'), 'type' => 'number', 'desc' => __('Enter a number', 'woocommerce'), 'id' => 'general_number_field' ), 'section_end' => array( 'type' => 'sectionend', 'id' => 'general_section_end' ), ); } } } return new WC_Settings_Custom();
Use the settings on the front end
Now, let’s use these settings on the front end of your WooCommerce site.
1) Get the value of the settings:
<?php $general_text_field = get_option('general_text_field'); $general_number_field = get_option('general_number_field'); $advanced_text_field = get_option('advanced_text_field');
2) Display the values on the front end (e.g., in the header.php
file or any other template):
<?php if ($general_text_field) : ?> <p><?php echo esc_html($general_text_field); ?></p> <p><?php echo esc_html($general_number_field); ?></p> <?php endif; ?> <?php if ($advanced_text_field) : ?> <p><?php echo esc_html($advanced_text_field); ?></p> <?php endif; ?>
Adding a custom tab to the WooCommerce settings page and dividing it into sections using the get_sections
method is an excellent way to organize and extend your store’s functionality. By using the woocommerce_get_settings_pages
hook, you can create custom settings tailored to your specific needs and then use those values on the front end to offer a more personalized experience to your users.