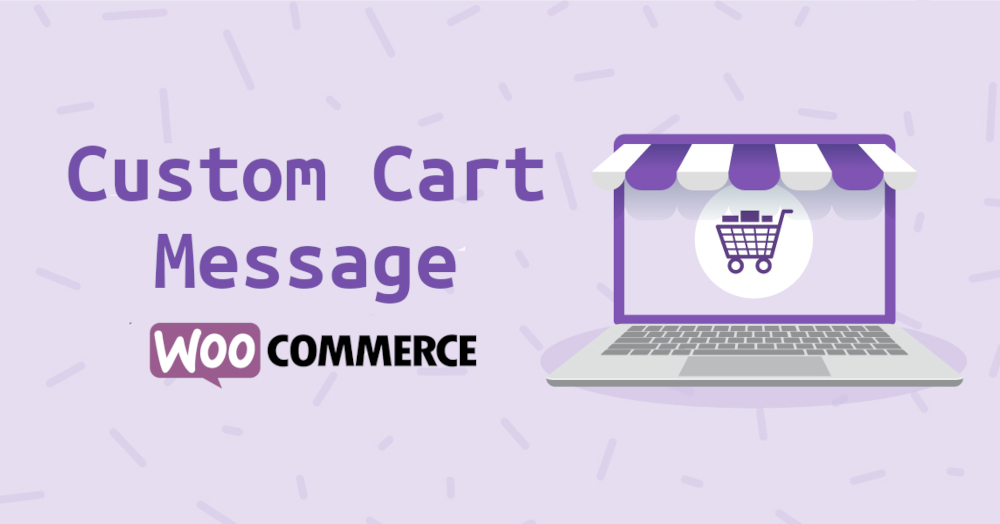
How to change the “Product Added to Cart” message in WooCommerce
WooCommerce is one of the most popular platforms for creating online stores with WordPress. One of the advantages of WooCommerce is its flexibility to customize almost any aspect of your store, including the messages displayed to customers. In this article, I’ll show you how to change the “Product Added to Cart” message using the wc_add_to_cart_message_html
hook.
What is the wc_add_to_cart_message_html Hook?
The wc_add_to_cart_message_html
hook allows you to modify the HTML of the message displayed when a product is added to the cart. This is useful if you want to customize this message to match your store’s style or to provide additional information to your customers.
Basic example
To start, let’s look at a basic example of how to change the default message. Add the following PHP code to your active theme’s functions.php
file.
<?php add_filter('wc_add_to_cart_message_html', 'custom_add_to_cart_message', 10, 2); function custom_add_to_cart_message($message, $products) { $new_message = "Product successfully added to the cart!"; return $new_message; }
In this example, we’ve changed the default message to “Product successfully added to the cart!”.
Example with product name
If you want to include the product name in the message, you can do it as follows:
<?php add_filter('wc_add_to_cart_message_html', 'custom_add_to_cart_message', 10, 2); function custom_add_to_cart_message($message, $products) { $product_names = array(); foreach ($products as $product_id => $qty) { $product = wc_get_product($product_id); $product_names[] = $product->get_name(); } $product_names_list = implode(', ', $product_names); $new_message = sprintf('You have added "%s" to the cart.', $product_names_list); return $new_message; }
This code retrieves the product name and includes it in the message.
Example with link to cart
You can also add a link to the cart for easier navigation for the user. Here’s how to do it:
<?php add_filter('wc_add_to_cart_message_html', 'custom_add_to_cart_message', 10, 2); function custom_add_to_cart_message($message, $products) { $product_names = array(); foreach ($products as $product_id => $qty) { $product = wc_get_product($product_id); $product_names[] = $product->get_name(); } $product_names_list = implode(', ', $product_names); $cart_url = wc_get_cart_url(); $new_message = sprintf('You have added "%s" to the cart. <a href="%s">View cart</a>.', $product_names_list, $cart_url); return $new_message; }
This example includes a link that takes the user to the shopping cart.
Example with different messages depending on the quantity of products
If you want to display a different message depending on the number of products added, you can use the following code:
<?php add_filter('wc_add_to_cart_message_html', 'custom_add_to_cart_message', 10, 2); function custom_add_to_cart_message($message, $products) { $product_names = array(); $total_quantity = 0; foreach ($products as $product_id => $qty) { $product = wc_get_product($product_id); $product_names[] = $product->get_name(); $total_quantity += $qty; } $product_names_list = implode(', ', $product_names); if ($total_quantity > 1) { $new_message = sprintf('You have added %d products (%s) to the cart.', $total_quantity, $product_names_list); } else { $new_message = sprintf('You have added "%s" to the cart.', $product_names_list); } return $new_message; }
In this case, a different message is displayed if the customer adds more than one product to the cart.
Customizing the “Add to Cart” message in WooCommerce is an excellent way to enhance the user experience and tailor messages to your store’s specific needs. Using the wc_add_to_cart_message_html
hook, you can modify this message in various ways according to your preferences.
I hope these examples are useful for customizing your WooCommerce store!