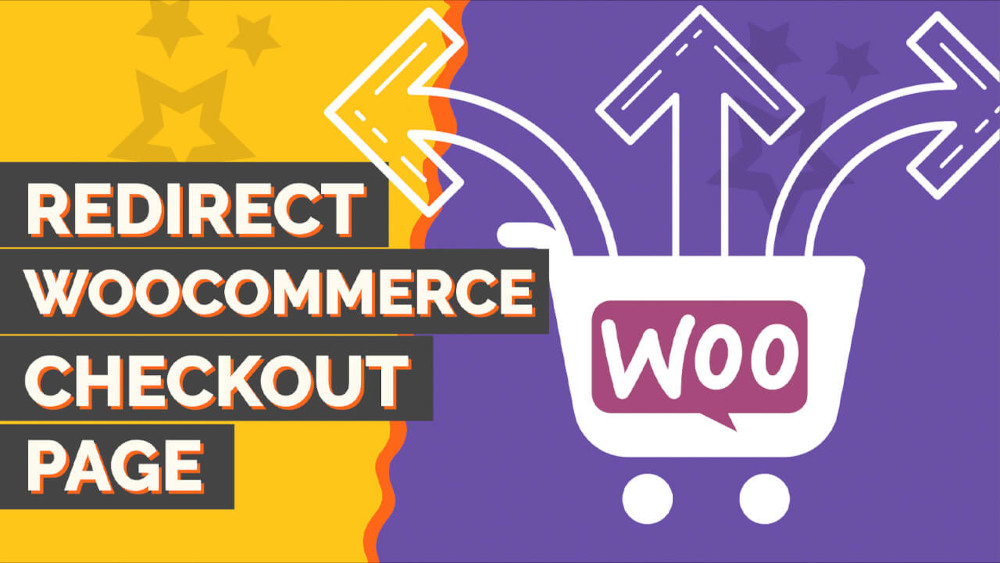
How to Redirect Users to the Checkout Page in WooCommerce
In WooCommerce, it can be beneficial to redirect users directly to the checkout page after they click “buy” on a product. This can improve the user experience by reducing the number of steps needed to complete a purchase. In this article, we will show you how to achieve this with some PHP code examples.
Add a hook for redirection.
To redirect users to the checkout page, we can use the woocommerce_add_to_cart_redirect
hook. This hook allows us to modify the redirect URL after a product is added to the cart.
First, add the following code to your theme’s functions.php
file:
<?php function custom_add_to_cart_redirect() { return wc_get_checkout_url(); } add_filter('woocommerce_add_to_cart_redirect', 'custom_add_to_cart_redirect');
custom_add_to_cart_redirect()
: This function defines the redirect URL. We usewc_get_checkout_url()
to get the URL of the checkout page.add_filter('woocommerce_add_to_cart_redirect', 'custom_add_to_cart_redirect')
: This filter applies our custom function to modify the redirect URL after a product is added to the cart.
Handle individual products.
If you want to redirect only for certain products, you can modify the function to check the product ID before redirecting. Here’s an example:
<?php function custom_add_to_cart_redirect($url) { // Get the ID of the product added to the cart $product_id = absint($_POST['add-to-cart']); // Specify the ID of the product you want to redirect $target_product_id = 123; // Change this to your product ID // Check if the product matches and redirect to the checkout page if ($product_id === $target_product_id) { $url = wc_get_checkout_url(); } return $url; } add_filter('woocommerce_add_to_cart_redirect', 'custom_add_to_cart_redirect');
absint($_POST['add-to-cart'])
: Gets the ID of the product that was added to the cart.123
: Replace this number with the specific product ID you want to redirect.- The condition
if ($product_id === $target_product_id)
checks if the added product ID matches the target product ID.
Redirect based on product category.
If you want to redirect based on the product category, you can modify the function to check the product’s category:
<?php function custom_add_to_cart_redirect_by_category($url) { // Get the ID of the product added to the cart $product_id = absint($_POST['add-to-cart']); // Get the product categories $product = wc_get_product($product_id); $product_categories = $product->get_category_ids(); // Specify the category you want to redirect $target_category_id = 10; // Change this to your category ID // Check if the product belongs to the target category and redirect to the checkout page if (in_array($target_category_id, $product_categories)) { $url = wc_get_checkout_url(); } return $url; } add_filter('woocommerce_add_to_cart_redirect', 'custom_add_to_cart_redirect_by_category');
wc_get_product($product_id)
: Gets the product object based on its ID.$product->get_category_ids()
: Gets the product’s categories as an array of IDs.10
: Replace this number with the category ID you want to redirect.
Redirecting users to the checkout page in WooCommerce can be an excellent way to enhance the shopping experience and increase conversions. With the above code examples, you can easily customize the redirection according to your specific needs, whether for all products, individual products, or products in certain categories.
I hope you found this article helpful! If you have any questions or need further assistance, feel free to leave a comment.