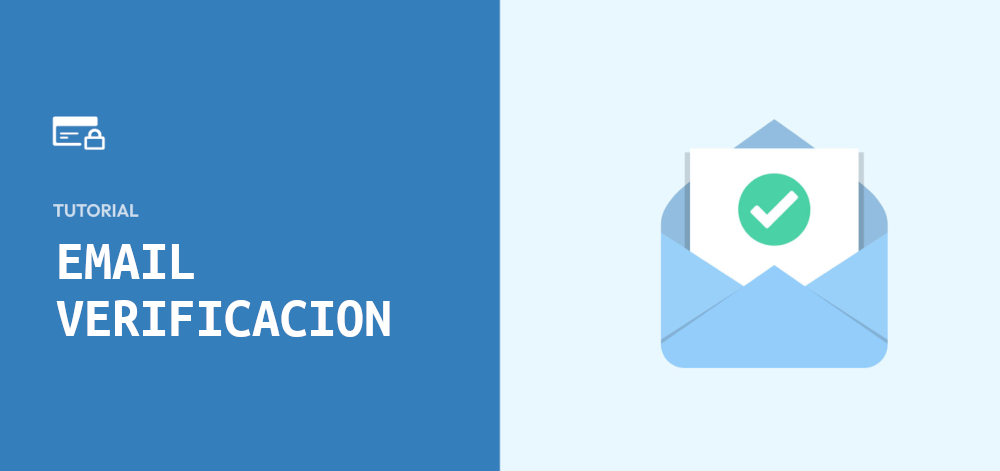
Implement email verification in user registration in WordPress
When managing a website with registered users, it’s crucial to ensure the email addresses provided are valid. Implementing an email verification process helps you avoid fake accounts and ensure the authenticity of your users. In this article, we’ll show you how to add an email verification process in WordPress.
1) Prepare the environment
Before you start, make sure you have access to your theme’s functions.php
file or work through a custom plugin to avoid issues during theme updates.
2) Modify the registration process
We need to hook a custom function to the WordPress registration process to send a verification email. We’ll add an additional field in the database to indicate whether the user has verified their email.
i. Add a verification field in the database:
<?php function add_user_verification_meta($user_id) { update_user_meta($user_id, 'is_email_verified', 0); } add_action('user_register', 'add_user_verification_meta');
ii. Send the verification email:
<?php function send_verification_email($user_id) { $user = get_userdata($user_id); $email = $user->user_email; $verification_code = md5(time()); // Save the verification code in the user's metadata update_user_meta($user_id, 'email_verification_code', $verification_code); // Create the verification link $verification_link = add_query_arg( array( 'user_id' => $user_id, 'verification_code' => $verification_code ), home_url('/verify-email/') ); // Send the email $subject = 'Verify Your Email Address'; $message = 'Please click the following link to verify your email address: ' . $verification_link; wp_mail($email, $subject, $message); } add_action('user_register', 'send_verification_email');
3) Create the verification page
We need a page where the user can click the verification link. We can use a custom page template to handle this.
i. Create the page template:
Create a file named page-verify-email.php
in your theme directory and add the following code:
<?php /* Template Name: Verify Email */ get_header(); if (isset($_GET['user_id']) && isset($_GET['verification_code'])) { $user_id = intval($_GET['user_id']); $verification_code = sanitize_text_field($_GET['verification_code']); $stored_code = get_user_meta($user_id, 'email_verification_code', true); if ($verification_code === $stored_code) { update_user_meta($user_id, 'is_email_verified', 1); delete_user_meta($user_id, 'email_verification_code'); echo '<p>Your email has been successfully verified!</p>'; } else { echo '<p>Invalid verification code.</p>'; } } else { echo '<p>Invalid request.</p>'; } get_footer(); ?>
ii. Create the Page in WordPress:
Go to Pages > Add New in the WordPress admin panel and create a new page called “Verify Email”. Assign the “Verify Email” template to this page from the page attributes panel.
4) Restrict access for unverified users
Finally, we need to ensure that users who have not verified their email cannot access certain areas of the site.
i. Add a verification check on login:
<?php function restrict_unverified_users($user_login, $user) { $is_verified = get_user_meta($user->ID, 'is_email_verified', true); if ($is_verified != 1) { wp_logout(); wp_redirect(home_url('/verification-required/')); exit(); } } add_action('wp_login', 'restrict_unverified_users', 10, 2);
ii. Create a notification page:
Create a new page in the WordPress admin panel called “Verification Required” with a message indicating that the user needs to verify their email to access the site.
Implementing email verification in WordPress improves the security and integrity of your user base. By following these steps, you can ensure that only users with valid email addresses can register and access your site. We hope this guide has been helpful!
Very helpful, works like a charm. Have you implemented a way to resend the email if the user needs it?