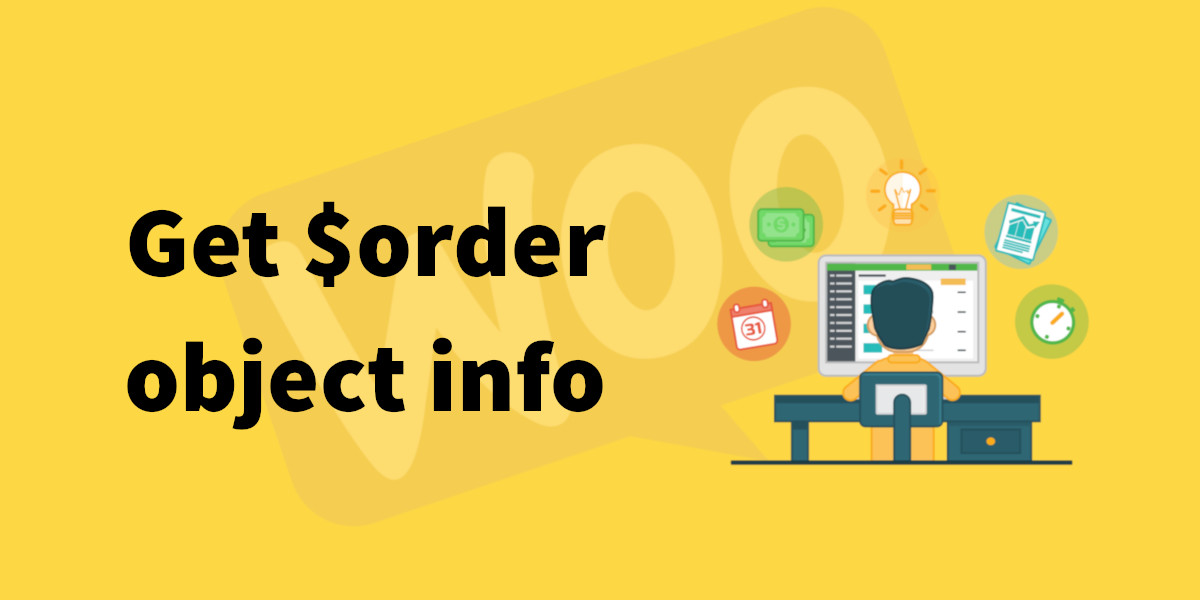
Get WooCommerce orders info from $order object
Sometimes we need to get information about an order and Woocommerce gives us many available methods for this purpose. Here we will explain each one of these methods and its use.
How to instance a $order object
First, you need to instance the Woocommerce order class.
<?php // If you have the Order ID $order = wc_get_order( $order_id ); // If you have the Order Key $order = wc_get_order_id_by_order_key( $order_key );
Methods with $order object
Now you have the $order object, you can call many methods. Here a description of each available method.
<?php // Get Order ID and Key $order->get_id(); $order->get_order_key(); // Get Order Status $order->get_status(); // Get tax $order->get_cart_tax(); // Get the currency code $order->get_currency(); // Get discount $order->get_discount_tax(); $order->get_discount_to_display(); $order->get_discount_total(); // Get Taxes $order->get_fees(); // Get Shipping Taxes $order->get_shipping_tax(); $order->get_shipping_total(); // Get Order Totals $order->get_subtotal(); $order->get_subtotal_to_display(); $order->get_tax_location(); $order->get_tax_totals(); $order->get_formatted_line_subtotal(); $order->get_formatted_order_total(); $order->get_total(); $order->get_total_discount(); $order->get_total_tax(); // Get Taxes $order->get_taxes(); // Get Refund Data $order->get_total_refunded(); $order->get_total_tax_refunded(); $order->get_total_shipping_refunded(); $order->get_item_count_refunded(); $order->get_total_qty_refunded(); $order->get_qty_refunded_for_item(); $order->get_total_refunded_for_item(); $order->get_tax_refunded_for_item(); $order->get_total_tax_refunded_by_rate_id(); $order->get_remaining_refund_amount(); // Get Items foreach ( $order->get_items() as $item_id => $item ) { // Get Product ID $product_id = $item->get_product_id(); // Get Variation ID $variation_id = $item->get_variation_id(); // Get Product Data $product = $item->get_product(); // Get Product name $name = $item->get_name(); // Get qty $quantity = $item->get_quantity(); // Get Subtotal $subtotal = $item->get_subtotal(); // Get Total $total = $item->get_total(); // Get Taxes $tax = $item->get_subtotal_tax(); $taxclass = $item->get_tax_class(); $taxstat = $item->get_tax_status(); // Get all the meta values $allmeta = $item->get_meta_data(); // Get the value of the meta called _whatever $somemeta = $item->get_meta( '_whatever', true ); // Get Item type ( products | shipping | coupon ) $type = $item->get_type(); } // Get Items info $order->get_items_key(); $order->get_items_tax_classes(); $order->get_item_count(); $order->get_item_total(); $order->get_downloadable_items(); // Get Order Lines $order->get_line_subtotal(); $order->get_line_tax(); $order->get_line_total(); // Get Order Shipping $order->get_shipping_method(); $order->get_shipping_methods(); $order->get_shipping_to_display(); // Get Order Dates $order->get_date_created(); $order->get_date_modified(); $order->get_date_completed(); $order->get_date_paid(); // Get Order Customer Data $order->get_customer_id(); $order->get_user_id(); $order->get_user(); $order->get_customer_ip_address(); $order->get_customer_user_agent(); $order->get_created_via(); $order->get_customer_note(); $order->get_address_prop(); // Get Billing Info $order->get_billing_first_name(); $order->get_billing_last_name(); $order->get_billing_company(); $order->get_billing_address_1(); $order->get_billing_address_2(); $order->get_billing_city(); $order->get_billing_state(); $order->get_billing_postcode(); $order->get_billing_country(); $order->get_billing_email(); $order->get_billing_phone(); $order->get_formatted_billing_full_name(); $order->get_formatted_billing_address(); // Get Shipping Info $order->get_shipping_first_name(); $order->get_shipping_last_name(); $order->get_shipping_company(); $order->get_shipping_address_1(); $order->get_shipping_address_2(); $order->get_shipping_city(); $order->get_shipping_state(); $order->get_shipping_postcode(); $order->get_shipping_country(); $order->get_address(); $order->get_shipping_address_map_url(); $order->get_formatted_shipping_full_name(); $order->get_formatted_shipping_address(); // Get Order Payment Details $order->get_payment_method(); $order->get_payment_method_title(); $order->get_transaction_id(); // Get Order URLs $order->get_checkout_payment_url(); $order->get_checkout_order_received_url(); $order->get_cancel_order_url(); $order->get_cancel_order_url_raw(); $order->get_cancel_endpoint(); $order->get_view_order_url(); $order->get_edit_order_url();
Any questions in the comments