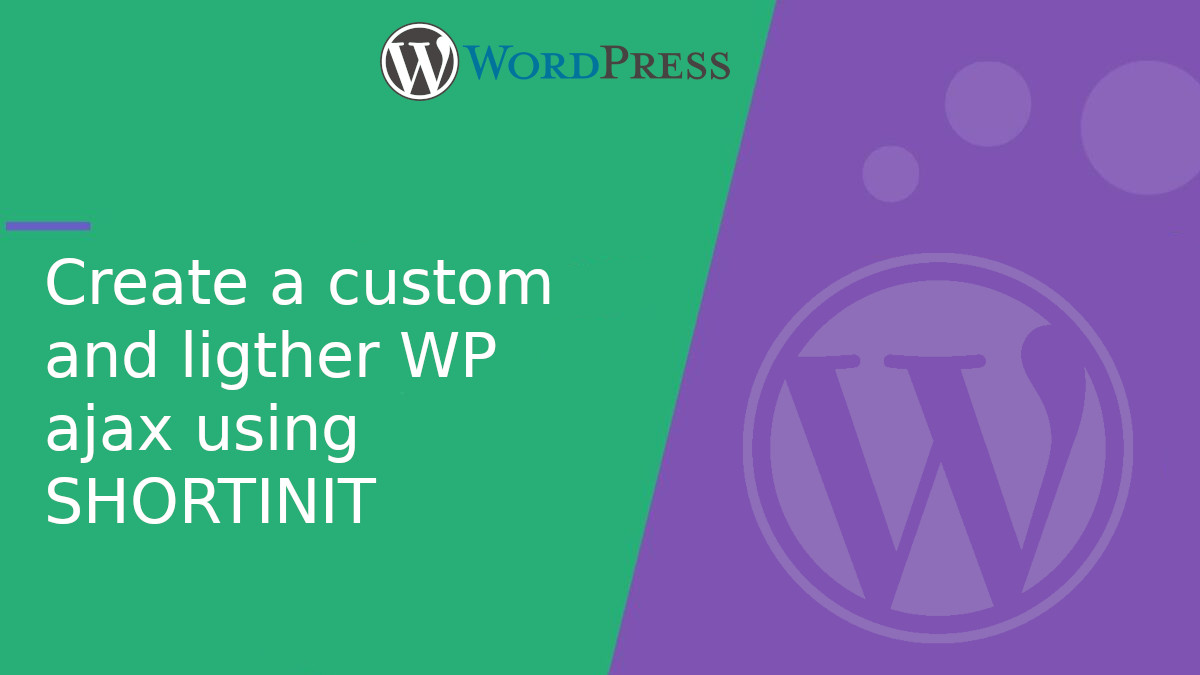
Create a custom and ligther WP ajax using SHORTINIT
WordPress provides admin-ajax.php
for handling AJAX requests, but it can be too heavy for specific applications as it loads the entire core. If you need something lighter, you can use SHORTINIT
. Below, we show you how to implement a custom file called custom-admin-ajax.php
.
What is SHORTINIT?
SHORTINIT
is a constant in WordPress that limits the functionalities loaded. When set to true
, only the following elements are loaded:
- The
wp-load.php
file. - Basic WordPress functions like
wpdb
,wp_safe_redirect
, andsanitize_text_field
. - The database connection and essential utilities for working with data.
This makes requests much faster by avoiding the full WordPress system, including plugins and themes.
Create the custom WP Ajax file
Create a file named custom-admin-ajax.php
in the wp-admin/
directory alongside admin-ajax.php
.
<?php define( 'SHORTINIT', true ); require_once __DIR__ . '/../wp-load.php'; // Check if an action is passed. if ( empty( $_REQUEST['action'] ) ) { wp_die( '0', 400 ); } $action = sanitize_text_field( $_REQUEST['action'] ); if ( has_action( "wp_custom_ajax_{$action}" ) ) { do_action( "wp_custom_ajax_{$action}" ); } else { wp_die( '0', 400 ); } // If nothing is output, end with a default message. wp_die( '0' );
Setup in the active theme
In the functions.php
file of the active theme, we’ll register the JavaScript file, the AJAX event, and a shortcode to display the HTML button.
Register the script and AJAX event
<?php function enqueueCustomAjaxScript() { wp_enqueue_script( 'custom-ajax-script', get_template_directory_uri() . '/custom-ajax.js', [], '1.0', true ); wp_localize_script( 'custom-ajax-script', 'customAjax', [ 'url' => admin_url( 'custom-admin-ajax.php' ), ] ); } add_action( 'wp_enqueue_scripts', 'enqueueCustomAjaxScript' );
Handle the action in PHP
Define the custom action to process the AJAX request and return the local and UTC time.
<?php function handleShowTime() { $localTime = current_time( 'H:i:s' ); $utcTime = gmdate( 'H:i:s' ); wp_send_json_success( [ 'local_time' => $localTime, 'utc_time' => $utcTime, ] ); } add_action( 'wp_custom_ajax_show_time', 'handleShowTime' );
Shortcode to display the button
<?php function renderAjaxButton() { return '<button id="show-time-btn">Show Time</button><div id="time-output"></div>'; } add_shortcode( 'show_time_button', 'renderAjaxButton' );
Create the JavaScript file
Create a file named custom-ajax.js
in your theme directory with the following content:
document.addEventListener('DOMContentLoaded', function() { const button = document.getElementById('show-time-btn'); const output = document.getElementById('time-output'); button.addEventListener('click', function() { fetch(customAjax.url + '?action=show_time') .then(function(response) { return response.json(); }) .then(function(data) { if (data.success) { output.innerHTML = '<p>Local Time: ' + data.data.local_time + '</p>' + '<p>UTC Time: ' + data.data.utc_time + '</p>'; } else { output.innerHTML = '<p>Error: ' + data.data.message + '</p>'; } }) .catch(function(error) { output.innerHTML = '<p>Connection Error: ' + error.message + '</p>'; }); }); });
Result
- Add the shortcode
[show_time_button]
to any post or page. - When the page loads, you will see a button labeled “Show Time.”
- Clicking the button will display the local and UTC time using our custom AJAX admin.
With this setup, you can handle AJAX requests more efficiently and lightly in WordPress. This approach is ideal for applications where performance is crucial, and only the basic core functionalities are needed.