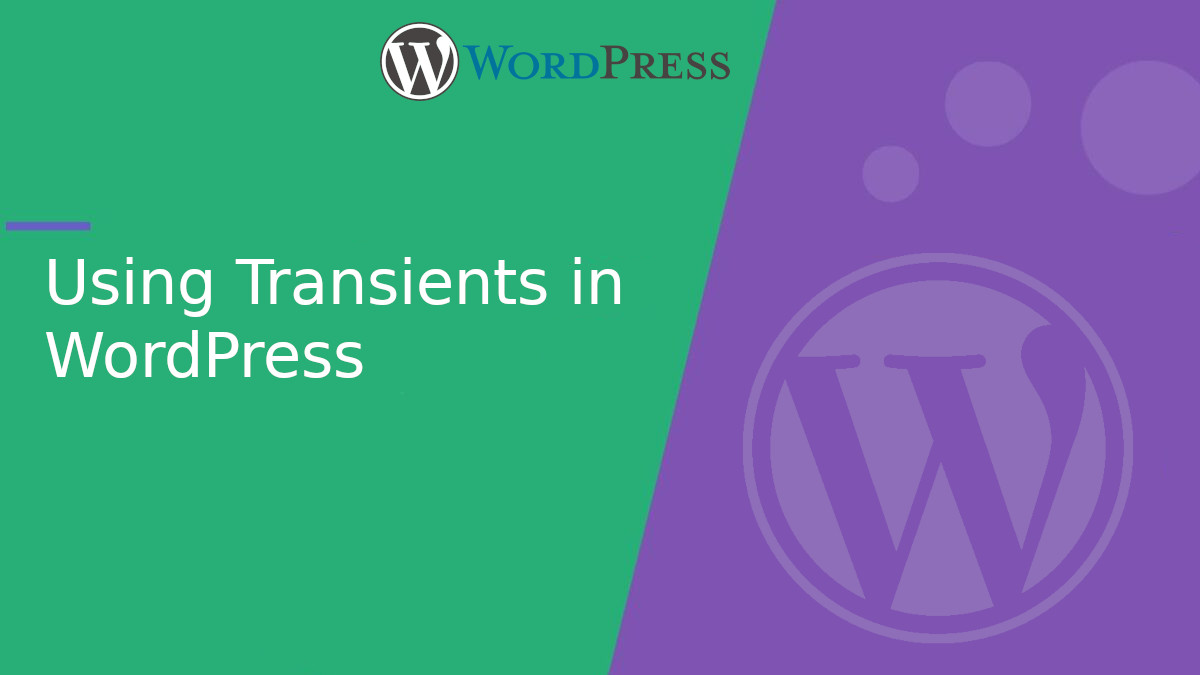
Using Transients in WordPress
Transients are a powerful tool in WordPress that allow you to temporarily cache data. Their main purpose is to reduce the execution time of complex or resource-intensive processes, such as heavy SQL queries or API requests. In this article, we will explore how transients work, practical use cases with PHP examples, and how they can significantly enhance your site’s performance.
What are transients?
A transient in WordPress is a form of temporary storage in the database. Each transient has:
- A unique key: To identify the stored data.
- A value: The information to be stored.
- An expiration time: The duration in seconds for which the data is valid.
When the configured time expires, the data is automatically removed.
Advantages of using transients
- Reduced database load: Expensive queries can be executed once and stored for reuse.
- Improved performance: Less time processing complex operations.
- Easy implementation: WordPress provides simple functions to manage them.
Main functions for working with transients
1. set_transient()
Stores data temporarily.
<?php set_transient( 'transientKey', 'valueToStore', 3600 );
2. get_transient()
Retrieves stored data if it hasn’t expired yet.
<?php $value = get_transient( 'transientKey' ); if ( $value ) { // Data retrieved from cache } else { // No cached data available }
3. delete_transient()
Manually deletes a transient.
<?php delete_transient( 'transientKey' );
Practical Example: listing top-selling products using SQL
Suppose you have a costly query to retrieve the best-selling products from a WooCommerce store. Using transients can prevent running the same query repeatedly.
<?php function getTopSellingProducts() { global $wpdb; // Attempt to get data from cache $topSellingProducts = get_transient( 'topSellingProducts' ); if ( ! $topSellingProducts ) { // Run the query if no cached data is found $topSellingProducts = $wpdb->get_results( "SELECT post_id, meta_value FROM {$wpdb->prefix}postmeta WHERE meta_key = '_sales' ORDER BY meta_value DESC LIMIT 10" ); // Store the result in a transient for 12 hours (43200 seconds) set_transient( 'topSellingProducts', $topSellingProducts, 43200 ); } return $topSellingProducts; }
Advantages of using transients
- The SQL query runs only when the transient expires.
- Subsequent requests to retrieve top-selling products fetch data from the cache, reducing database load.
How they are stored in the database
Transients are stored in WordPress’s options table (wp_options
by default). When you create a transient, WordPress generates two entries:
- One for the transient value (
_transient_[key]
). - Another for the expiration date (
_transient_timeout_[key]
).
If you use a caching system like Memcached or Redis, transients will be stored there instead, bypassing the database and offering even better performance.
Important considerations
- Storage space: Transients are stored in the database by default, but with a caching system like Memcached or Redis, they are stored there for improved performance.
- Expiration control: Use a reasonable expiration time depending on how frequently the data changes.
- Manual deletion: If a transient contains data sensitive to changes, remember to delete it manually when an update occurs.
<?php add_action( 'save_post', function( $postId ) { delete_transient( 'topSellingProducts' ); } );
Transients are an efficient solution to enhance the performance of your WordPress site, especially when working with repetitive operations or costly queries. Implementing them is straightforward, and the benefits in speed and user experience are remarkable. Start using them today to optimize your site!