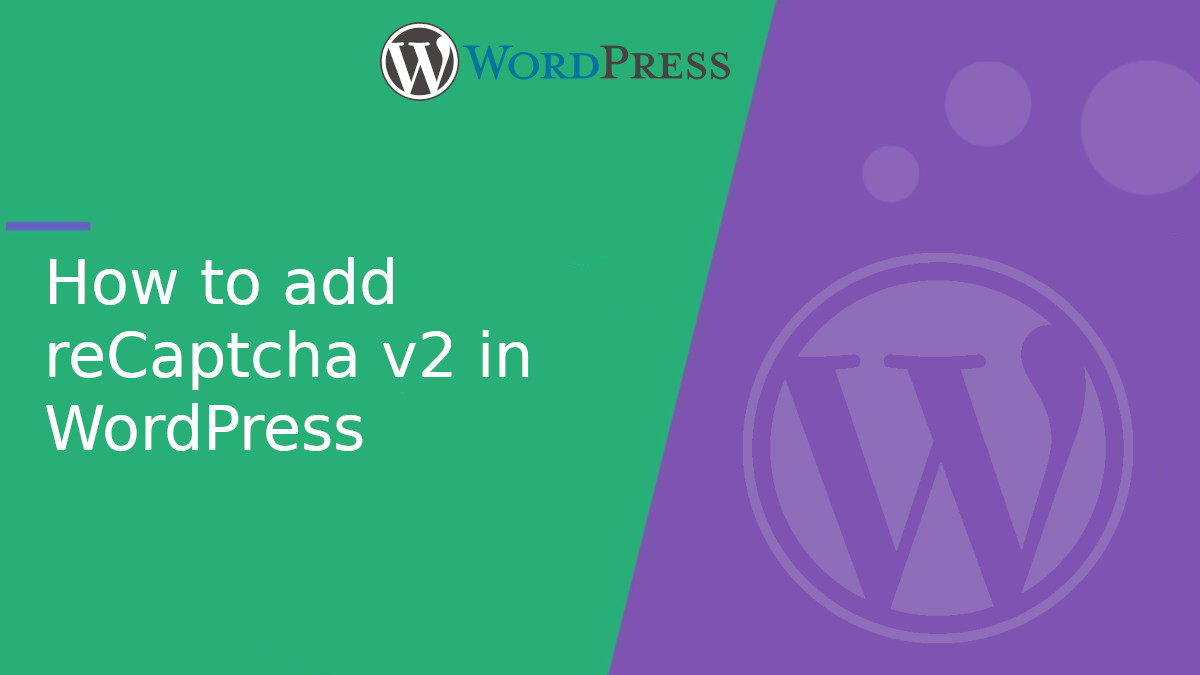
How to add reCaptcha V2 in a custom form in WordPress
Security is crucial for any website, and Google reCAPTCHA is an excellent tool to prevent spam and automated submissions. In this article, you’ll learn how to implement reCAPTCHA v2 on WordPress with a simple custom form, using only code snippets.
Get Your reCAPTCHA Keys
- Go to Google reCAPTCHA.
- Register your site and select the reCAPTCHA v2 option.
- Obtain the Site Key and Secret Key. Save them as you’ll need them later.
Create the Basic Form
Let’s create a simple form that includes the reCAPTCHA. Use the following snippet to generate a form with a shortcode.
<?php // Create the form with reCAPTCHA v2 function custom_recaptcha_form() { $siteKey = 'YOUR_SITE_KEY'; // Replace with your Site Key ob_start(); ?> <form id="customForm" method="post" action=""> <p> <label for="name">Name</label> <input type="text" name="name" id="name" required> </p> <p> <label for="email">Email</label> <input type="email" name="email" id="email" required> </p> <div class="g-recaptcha" data-sitekey="<?php echo esc_attr( $siteKey ); ?>"></div> <p> <input type="submit" name="submit" value="Submit"> </p> </form> <?php return ob_get_clean(); } add_shortcode('custom_recaptcha_form', 'custom_recaptcha_form');
Add the shortcode [custom_recaptcha_form]
to any page to display the form.
Load the reCAPTCHA Script
You need to include the reCAPTCHA script on the page. Use this snippet to load it:
<?php // Load the reCAPTCHA script in the frontend function load_recaptcha_script() { wp_enqueue_script( 'google-recaptcha', 'https://www.google.com/recaptcha/api.js', [], null, true ); } add_action('wp_enqueue_scripts', 'load_recaptcha_script');
Validate reCAPTCHA on the Server
To verify the user isn’t a bot, send the token generated by reCAPTCHA to the server and validate it using the secret key. Use the following code:
<?php // Validate reCAPTCHA on form submission function validate_recaptcha_submission() { if ( isset( $_POST['submit'] ) ) { $secretKey = 'YOUR_SECRET_KEY'; // Replace with your Secret Key $recaptchaResponse = sanitize_text_field( $_POST['g-recaptcha-response'] ); $remoteIp = $_SERVER['REMOTE_ADDR']; $response = wp_remote_post( 'https://www.google.com/recaptcha/api/siteverify', [ 'body' => [ 'secret' => $secretKey, 'response' => $recaptchaResponse, 'remoteip' => $remoteIp, ], ]); $responseBody = json_decode( wp_remote_retrieve_body( $response ), true ); if ( ! $responseBody['success'] ) { wp_die('reCAPTCHA verification failed. Please try again.'); } // Process form data if validation is successful echo '<p>Form submitted successfully!</p>'; } } add_action('init', 'validate_recaptcha_submission');
Customize Validation and Design (Optional)
You can add custom styles to improve the form’s appearance. For example:
<?php // Styles for the form function custom_form_styles() { echo '<style> form#customForm { max-width: 400px; margin: 0 auto; padding: 20px; border: 1px solid #ddd; border-radius: 5px; background-color: #f9f9f9; } form#customForm p { margin-bottom: 15px; } form#customForm input { width: 100%; padding: 10px; border: 1px solid #ccc; border-radius: 5px; } </style>'; } add_action('wp_head', 'custom_form_styles');
Summary
- Insert the shortcode
[custom_recaptcha_form]
into a page to display the form. - Submit the form to verify that reCAPTCHA correctly blocks automated requests.
- If you need to disable the form on certain pages, wrap the shortcode in a conditional statement within the code.