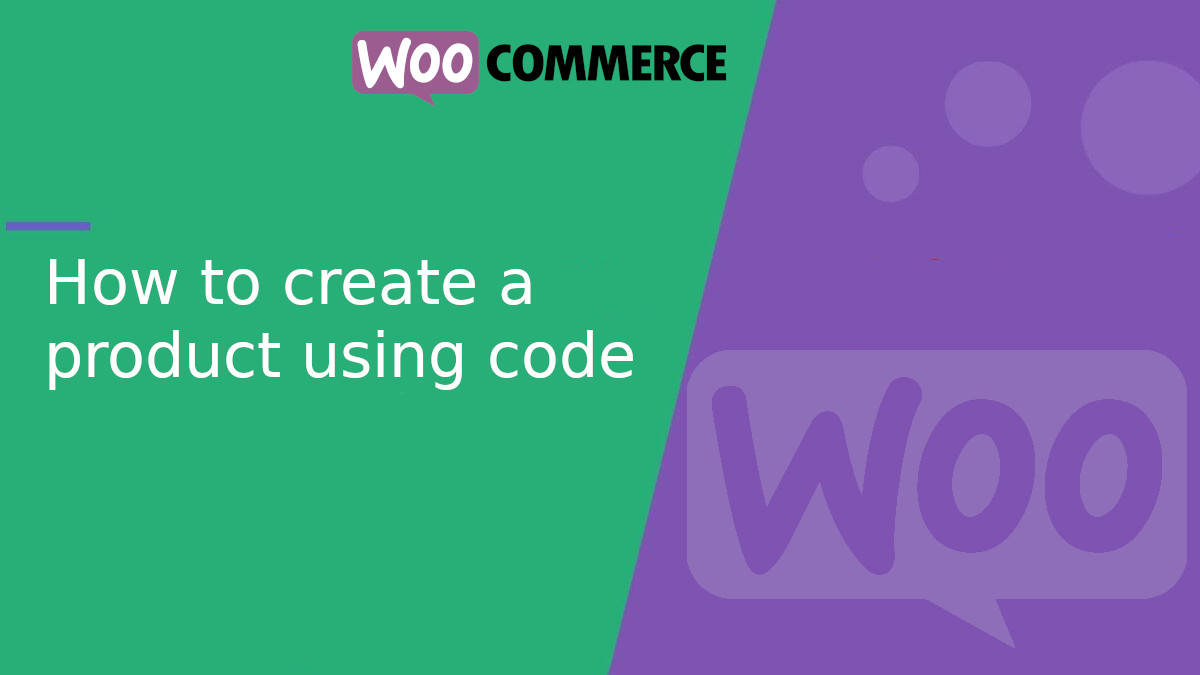
How to create a product in WooCommerce using code
WooCommerce allows us to manually add products from the WordPress admin panel, but we can also create them programmatically using code. This is useful when automating product creation, importing bulk data, or customizing the store with personalized scripts.
In this article, we will see how to create three different types of products:
- Simple Product
- Variable Product
- External/Affiliate Product
Creating a simple product
A simple product is the most basic type, without variations.
<?php function createSimpleProduct() { $product = new WC_Product_Simple(); $product->set_name('Simple Product'); $product->set_description('This is a simple product created with code.'); $product->set_price(50); $product->set_regular_price(50); $product->set_stock_status('instock'); $productId = $product->save(); } add_action('init', 'createSimpleProduct');
Creating a variable product
A variable product has different variations with distinct attributes.
<?php function createVariableProduct() { $product = new WC_Product_Variable(); $product->set_name('Variable Product'); $product->set_description('This product has variations.'); $product->set_stock_status('instock'); $productId = $product->save(); $attribute = new WC_Product_Attribute(); $attribute->set_name('Color'); $attribute->set_options(['Red', 'Blue', 'Green']); $attribute->set_visible(true); $attribute->set_variation(true); $product->set_attributes([$attribute]); $product->save(); } add_action('init', 'createVariableProduct');
Creating an external/affiliate product
An external product is not sold directly in the store but redirects to another website.
<?php function createExternalProduct() { $product = new WC_Product_External(); $product->set_name('External Product'); $product->set_description('This is an affiliate product.'); $product->set_product_url('https://example.com/product'); $product->set_button_text('Buy on Example'); $productId = $product->save(); } add_action('init', 'createExternalProduct');
These examples demonstrate how to create different types of products in WooCommerce using code according to the WooCommerce Codex. You can customize them according to your needs by adding more metadata or integrating them into automated processes.