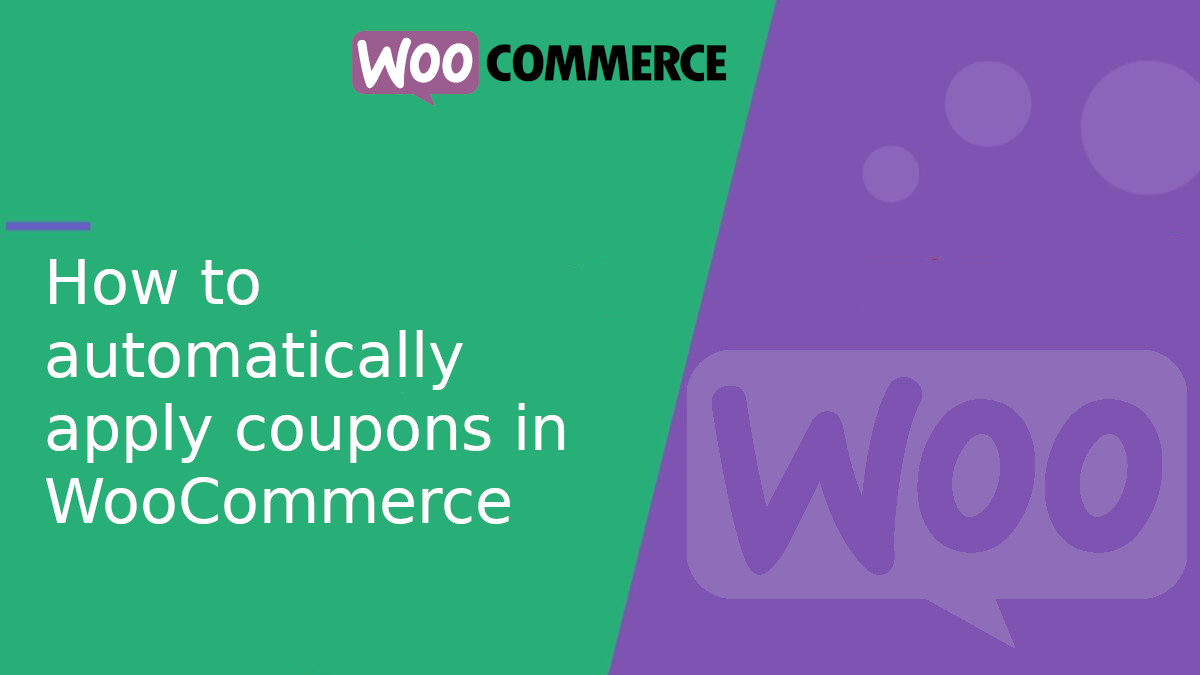
How to automatically apply coupons in WooCommerce
Coupons are a great way to boost sales in a WooCommerce store. However, asking customers to manually enter a code can create unnecessary friction in the purchasing process. In this article, we will show you how to automatically apply coupons in WooCommerce using PHP code.
Before you begin: create the coupon in WooCommerce
Before applying a coupon automatically, you must first create it manually in WooCommerce. To do this:
- Go to WooCommerce > Marketing > Coupons.
- Click on Add coupon.
- Enter a coupon code (e.g.,
DISCOUNT10
). - Set the discount and restrictions according to your needs.
- Save the changes.
Once the coupon is created, we can apply it automatically with code.
Methods to automatically apply coupons
There are different scenarios in which we might want to apply a coupon automatically:
- When a user adds a specific product to the cart.
- When the cart reaches a minimum purchase amount.
- When the user accesses the store via a special URL parameter.
- Apply a coupon automatically across the entire store.
Let’s see how to implement each of these methods.
1. Apply a coupon when a product is added to the cart
If we want to apply a coupon when the user adds a specific product, we can use the woocommerce_add_to_cart
hook.
<?php function applyCouponOnAddToCart( $cartItemKey, $productId, $quantity, $variationId, $cart ) { $couponCode = 'DISCOUNT10'; // Replace with your coupon if ( ! $cart->has_discount( $couponCode ) ) { $cart->apply_coupon( $couponCode ); } } add_action( 'woocommerce_add_to_cart', 'applyCouponOnAddToCart', 10, 5 );
Explanation
- This code checks if the coupon has not yet been applied and adds it when a product is added to the cart.
2. Apply a coupon if the cart total exceeds a certain amount
If we want to apply the coupon when the cart reaches a certain amount, we can use the woocommerce_before_calculate_totals
hook.
<?php function applyCouponOnCartTotal( $cart ) { if ( is_admin() && ! defined( 'DOING_AJAX' ) ) { return; } $couponCode = 'DISCOUNT15'; // Replace with your coupon $minimumTotal = 100; // Minimum cart total in store currency if ( $cart->subtotal >= $minimumTotal && ! $cart->has_discount( $couponCode ) ) { $cart->apply_coupon( $couponCode ); } } add_action( 'woocommerce_before_calculate_totals', 'applyCouponOnCartTotal' );
Explanation
- If the cart subtotal exceed
$minimumTotal
, the coupon is applied automatically.
3. Apply a coupon when the user accesses a special URL
We can allow customers to activate a coupon through a special URL, such as:
https://yourdomain.com/shop/?apply_coupon=DISCOUNT20
To achieve this, we add the following code:
<?php function applyCouponFromUrl() { if ( isset( $_GET['apply_coupon'] ) ) { $couponCode = sanitize_text_field( $_GET['apply_coupon'] ); if ( WC()->cart && ! WC()->cart->has_discount( $couponCode ) ) { WC()->cart->apply_coupon( $couponCode ); wc_add_notice( 'Coupon successfully applied!', 'success' ); } } } add_action( 'wp_loaded', 'applyCouponFromUrl' );
Explanation
- Detects if the
apply_coupon
parameter is present in the URL and applies the coupon automatically. - Displays a store notice indicating that the coupon has been added.
4. Apply a coupon automatically to the entire store
If we want a coupon to be applied to every active cart, regardless of the products added or the cart total, we can use the following:
<?php function applyCouponOnEveryCart( $cart ) { if ( is_admin() && ! defined( 'DOING_AJAX' ) ) { return; } $couponCode = 'GLOBALDISCOUNT'; // Replace with your coupon if ( ! $cart->has_discount( $couponCode ) ) { $cart->apply_coupon( $couponCode ); } } add_action( 'woocommerce_before_calculate_totals', 'applyCouponOnEveryCart' );
Explanation
- Applies the coupon to all active carts without restrictions.
- If the coupon is already applied, it is not added again.
Automatically applying coupons in WooCommerce enhances user experience and can increase conversions. You can use these methods depending on your needs: