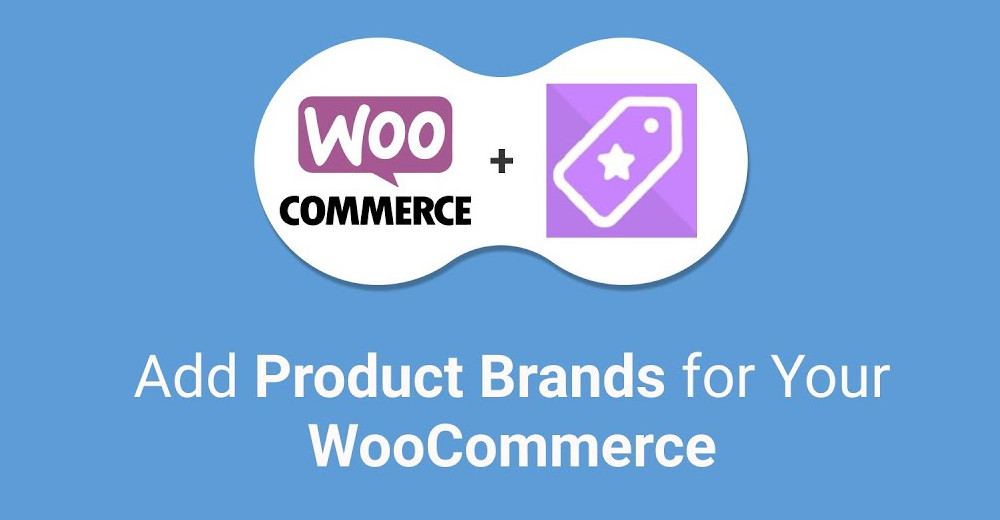
How to add Brands to WooCommerce products
In this article, we’ll show you how to add a custom taxonomy called “Brands” to your WooCommerce products. We’ll also show you how to add an image field to this taxonomy and how to create a shortcode to display these images with links to the products filtered by each brand.
Create the “Brands” Taxonomy
First, we need to register the new taxonomy “Brands.” To do this, add the following code to your theme’s functions.php
file:
<?php // Register custom taxonomy "Brands" function create_brand_taxonomy() { $labels = array( 'name' => _x('Brands', 'taxonomy general name', 'textdomain'), 'singular_name' => _x('Brand', 'taxonomy singular name', 'textdomain'), 'search_items' => __('Search Brands', 'textdomain'), 'all_items' => __('All Brands', 'textdomain'), 'parent_item' => __('Parent Brand', 'textdomain'), 'parent_item_colon' => __('Parent Brand:', 'textdomain'), 'edit_item' => __('Edit Brand', 'textdomain'), 'update_item' => __('Update Brand', 'textdomain'), 'add_new_item' => __('Add New Brand', 'textdomain'), 'new_item_name' => __('New Brand Name', 'textdomain'), 'menu_name' => __('Brands', 'textdomain'), ); $args = array( 'hierarchical' => true, 'labels' => $labels, 'show_ui' => true, 'show_admin_column' => true, 'query_var' => true, 'rewrite' => array('slug' => 'brand'), ); register_taxonomy('brand', array('product'), $args); } add_action('init', 'create_brand_taxonomy', 0);
This code creates a new hierarchical taxonomy called “Brands” that can be used to categorize products.
Add an image field to the “Brands” taxonomy
To add an image field to our “Brands” taxonomy, we can use the following code:
<?php // Add image field to "Brands" taxonomy function brand_add_image_field() { ?> <div class="form-field term-group"> <label for="brand_image"><?php _e('Brand Image', 'textdomain'); ?></label> <input type="text" id="brand_image" name="brand_image" value=""> <button class="upload_image_button button"><?php _e('Upload/Add Image', 'textdomain'); ?></button> </div> <?php } add_action('brand_add_form_fields', 'brand_add_image_field', 10, 2); // Save the image field function save_brand_image($term_id) { if (isset($_POST['brand_image']) && '' !== $_POST['brand_image']) { $image = esc_url($_POST['brand_image']); add_term_meta($term_id, 'brand_image', $image, true); } } add_action('created_brand', 'save_brand_image', 10, 2); // Edit the image field function brand_edit_image_field($term, $taxonomy) { $image = get_term_meta($term->term_id, 'brand_image', true); ?> <tr class="form-field term-group-wrap"> <th scope="row"><label for="brand_image"><?php _e('Brand Image', 'textdomain'); ?></label></th> <td> <input type="text" id="brand_image" name="brand_image" value="<?php echo esc_attr($image); ?>"> <button class="upload_image_button button"><?php _e('Upload/Add Image', 'textdomain'); ?></button> </td> </tr> <?php } add_action('brand_edit_form_fields', 'brand_edit_image_field', 10, 2); // Update the image field function update_brand_image($term_id) { if (isset($_POST['brand_image']) && '' !== $_POST['brand_image']) { $image = esc_url($_POST['brand_image']); update_term_meta($term_id, 'brand_image', $image); } } add_action('edited_brand', 'update_brand_image', 10, 2); // Enqueue the media script function enqueue_media_script() { if (isset($_GET['taxonomy']) && $_GET['taxonomy'] == 'brand') { wp_enqueue_media(); wp_enqueue_script('brand-image-upload', get_template_directory_uri() . '/js/brand-image-upload.js', array('jquery'), null, true); } } add_action('admin_enqueue_scripts', 'enqueue_media_script'); // JavaScript for uploading the image function brand_image_upload_script() { ?> <script> jQuery(document).ready(function($) { function uploadImage(button_class) { var _custom_media = true, _orig_send_attachment = wp.media.editor.send.attachment; $('body').on('click', button_class, function(e) { var button_id = '#' + $(this).attr('id'); var send_attachment_bkp = wp.media.editor.send.attachment; var button = $(button_id); _custom_media = true; wp.media.editor.send.attachment = function(props, attachment) { if (_custom_media) { button.prev().val(attachment.url); } else { return _orig_send_attachment.apply(button_id, [props, attachment]); } } wp.media.editor.open(button); return false; }); } uploadImage('.upload_image_button'); }); </script> <?php } add_action('admin_footer', 'brand_image_upload_script');
This code adds an image field to the creation and editing form of the “Brands” taxonomy, allowing you to upload and save images.
Create a shortcode to list Brand images
Finally, we create a shortcode to display the brand images with links to the products filtered by each brand:
<?php // Shortcode to display brand images function brand_images_shortcode() { $terms = get_terms(array( 'taxonomy' => 'brand', 'hide_empty' => false, )); if (!empty($terms) && !is_wp_error($terms)) { $output = '<div class="brand-images">'; foreach ($terms as $term) { $image = get_term_meta($term->term_id, 'brand_image', true); $term_link = get_term_link($term); if ($image) { $output .= '<div class="brand-image">'; $output .= '<a href="' . esc_url($term_link) . '">'; $output .= '<img src="' . esc_url($image) . '" alt="' . esc_attr($term->name) . '">'; $output .= '</a>'; $output .= '</div>'; } } $output .= '</div>'; } else { $output = __('No brands available.', 'textdomain'); } return $output; } add_shortcode('brand_images', 'brand_images_shortcode');
This shortcode [brand_images]
can be used on any page or post to display the brand images with links to the products filtered by each brand.
By following these steps, you’ve successfully added a custom “Brands” taxonomy to your WooCommerce products, including an image field for each brand, and created a shortcode to display these images. This will help you better organize your products and offer a more personalized shopping experience for your customers.
We hope this tutorial was helpful! If you have any questions, feel free to leave a comment.