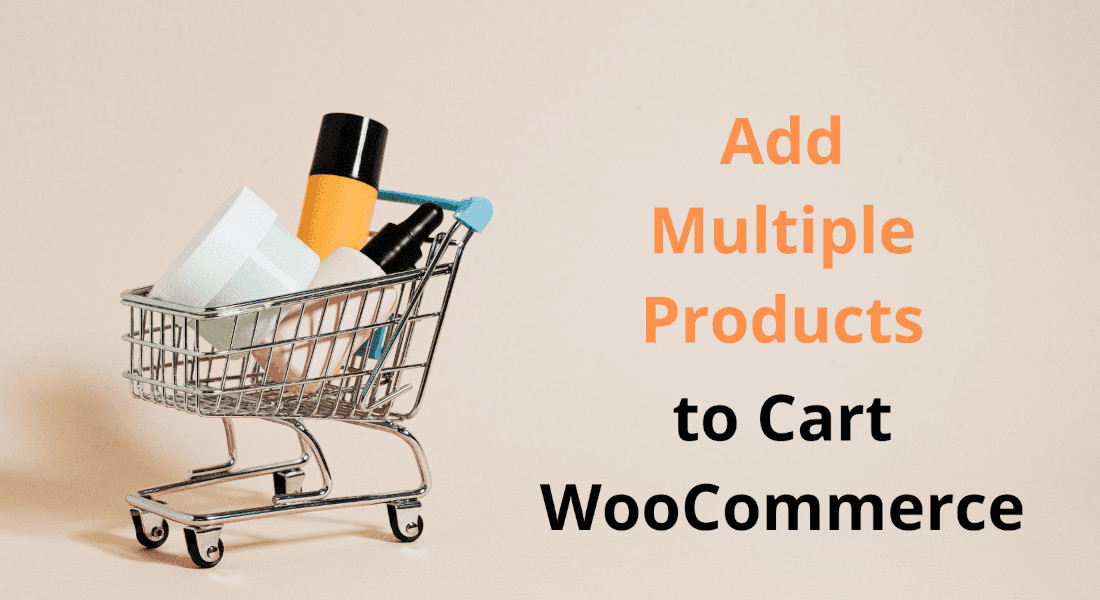
Add multiple products to cart by URL in WooCommerce
WooCommerce is a powerful tool for creating online stores, but sometimes the default functionalities are not enough for specific business needs. One example is the ability to add multiple products to the cart using a single link. By default, WooCommerce allows adding only one product at a time using the structure ?add-to-cart=ID
. In this article, we will show you how to modify this to accept multiple products separated by commas, like ?add-to-cart=1,2,3
.
Create a custom function
First, we will create a custom function that handles the logic of adding multiple products to the cart. This function will take the product IDs from the URL, separate them, and add them to the cart, as long as the variable contains commas.
<?php function addMultipleProductsToCart() { if ( isset($_REQUEST['add-to-cart']) ) { $addToCartParam = $_REQUEST['add-to-cart']; // Check if the parameter contains commas if ( strpos($addToCartParam, ',') !== false ) { $productIDs = explode(',', $addToCartParam); foreach ( $productIds as $productID ) { $productID = absint( $productID ); // Ensure the product ID is a positive integer if ( $productID > 0 ) { WC()->cart->add_to_cart( $productID ); } } // Redirect to the cart after adding the products wp_safe_redirect( wc_get_cart_url() ); exit; } } } add_action( 'init', 'addMultipleProductsToCart' );
Open the functions.php
file of your child theme and paste the above code at the end of the file or you can install a snippet PHP plugin and paste the code there.
Test the functionality
To test that everything works correctly, open your browser and use a URL similar to this:
https://yourwebsite.com/?add-to-cart=1,2,3
This should add the products with IDs 1, 2, and 3 to the cart and redirect the user to the cart page.
With these simple steps, you can extend WooCommerce functionality to allow users to add multiple products to the cart using a single link. This enhancement can be particularly useful in situations where you want to offer product bundles or make the purchasing process easier for your customers.
We hope this article has been helpful. If you have any questions or need further assistance, feel free to leave a comment.