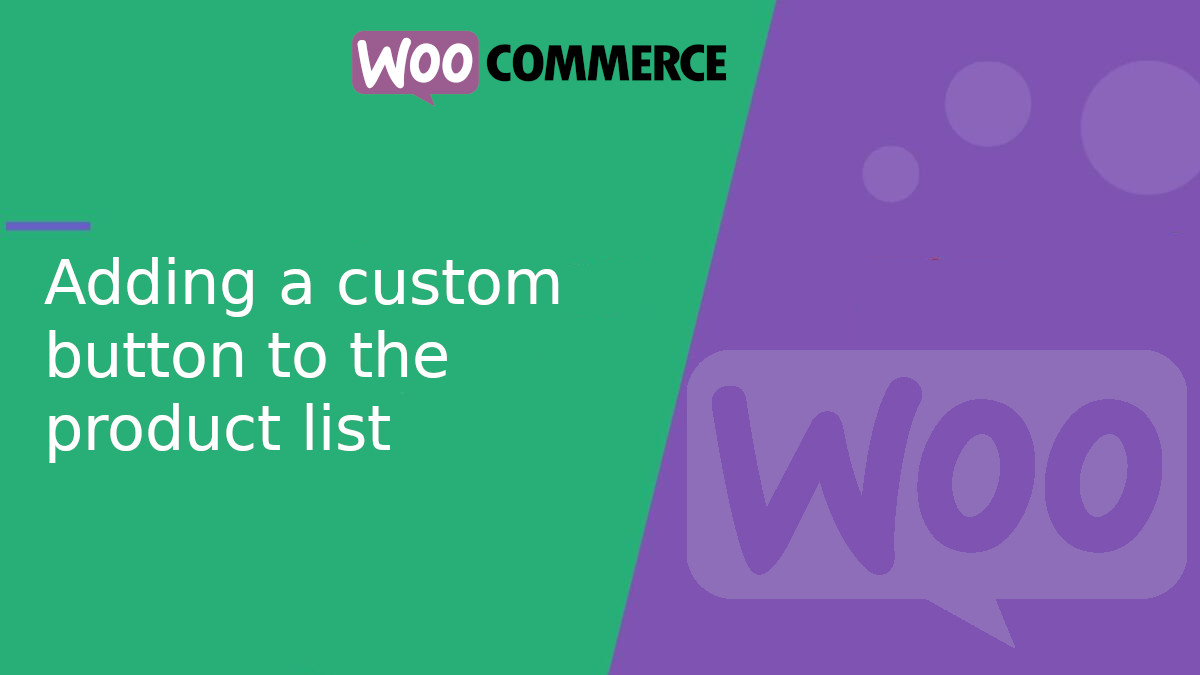
Adding a custom button to the product list in WooCommerce
In this article, I will show you how to add a custom button to the actions column in the WooCommerce product list. As an example, we will implement a button that, when clicked, sets the product stock to 0. If the product is variable, the stock of all its variations will also be updated to 0.
Adding the button to the actions column
In WooCommerce, we can modify the product list in the admin panel using the woocommerce_admin_product_actions
filter. We will use this filter to add our button.
<?php add_filter( 'woocommerce_admin_product_actions', function( $actions, $post ) { $url = wp_nonce_url( admin_url( "admin-ajax.php?action=zero_stock_product&product_id={$post->ID}" ), 'zero_stock_' . $post->ID ); $actions['zero_stock'] = [ 'url' => $url, 'name' => __( 'Set Stock to 0', 'woocommerce' ), 'action' => 'zero_stock_product', // Custom CSS classes ]; return $actions; }, 10, 2 );
Explanation:
- A new button is added to the actions column.
- A URL pointing to
admin-ajax.php
with thezero_stock_product
action is generated. wp_nonce_url()
is used to prevent unauthorized access.
Creating the function to process the action
Now, we need to handle the AJAX action triggered when clicking the button.
<?php add_action( 'wp_ajax_zero_stock_product', function() { if ( empty( $_GET['product_id'] ) || ! wp_verify_nonce( $_GET['_wpnonce'], 'zero_stock_' . $_GET['product_id'] ) ) { wp_die( __( 'Access not allowed', 'woocommerce' ) ); } $product_id = intval( $_GET['product_id'] ); $product = wc_get_product( $product_id ); if ( ! $product ) { wp_die( __( 'Product not found', 'woocommerce' ) ); } if ( $product->is_type( 'variable' ) ) { $variations = $product->get_children(); foreach ( $variations as $variation_id ) { $variation = wc_get_product( $variation_id ); if ( $variation ) { $variation->set_stock_quantity( 0 ); $variation->set_stock_status( 'outofstock' ); $variation->save(); } } } $product->set_stock_quantity( 0 ); $product->set_stock_status( 'outofstock' ); $product->save(); wp_safe_redirect( wp_get_referer() ); exit; });
Explanation:
- The
nonce
is validated to prevent unauthorized access. - The product ID is retrieved and checked for validity.
- If the product is variable, each variation is retrieved and its stock is updated.
- The stock of the main product is updated.
- The user is redirected back to the product list after execution.
Adding styles for the button
We can add some CSS to highlight our button in the WooCommerce interface:
- The button color is changed to red to indicate that it is an important action.
After implementing this code, you will see a new button called “Set Stock to 0” in the actions column of the WooCommerce product list. When clicked, the stock of the product (or its variations) will automatically be updated to 0.