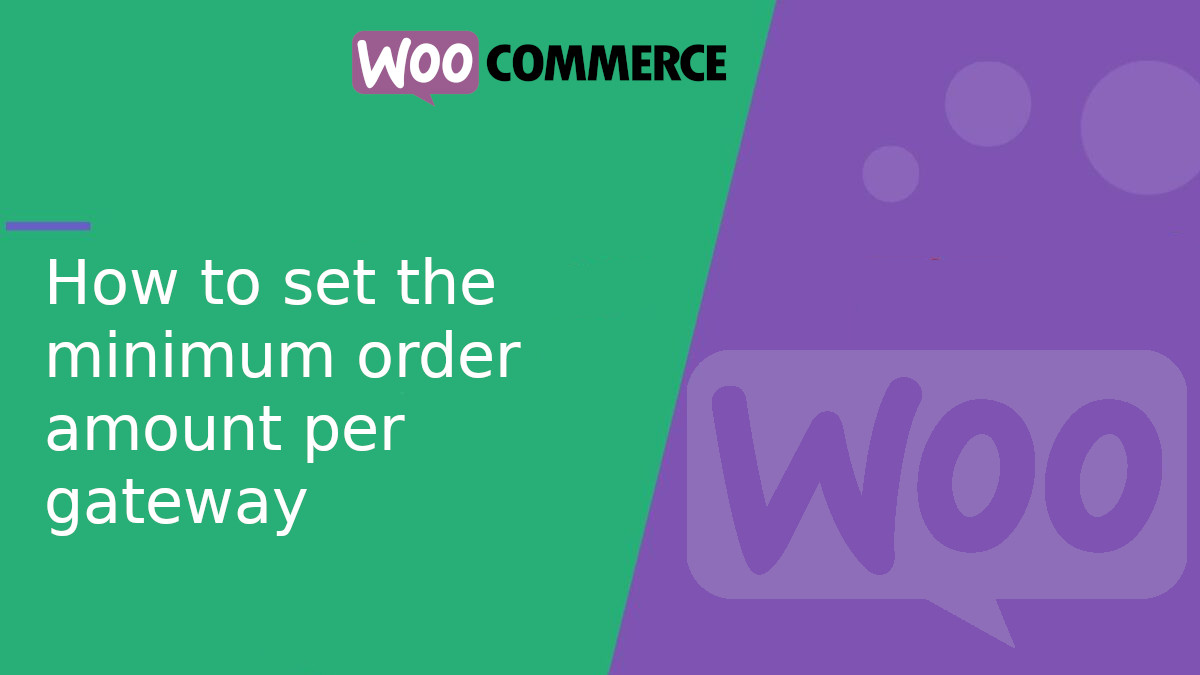
How to set the minimun order amount per gateway
WooCommerce allows you to set custom rules to optimize your store’s operations. In this case, you’ll learn how to implement a minimum order amount based on the selected payment gateway. We’ll achieve this in two steps:
Display a minimum order notification in the checkout
This step displays a notification on the order review page, indicating the minimum order amounts required for each payment gateway.
<?php function displayMinimumOrderNotification() { $paymentGatewayMinimums = [ 'paypal' => 20, 'cod' => 30, 'bacs' => 25, ]; $cartTotal = WC()->cart->total; echo '<div class="woocommerce-info">'; foreach ( $paymentGatewayMinimums as $gatewayId => $minimumAmount ) { if ( $cartTotal < $minimumAmount ) { printf( esc_html__( 'If you choose %s, your order total must be at least %s.', 'letsgo' ), ucfirst( $gatewayId ), wc_price( $minimumAmount ) ); echo '<br>'; } } echo '</div>'; } add_action( 'woocommerce_review_order_before_payment', 'displayMinimumOrderNotification' );
Explanation:
- The
$paymentGatewayMinimums
array defines the minimum amounts for each available payment method. - If the cart total is less than the required minimum, an informational message is displayed.
Prevent checkout if the minimum is not met
In this step, we prevent customers from completing the checkout if the cart total does not meet the minimum amount for the selected payment gateway. If no payment gateway is selected, a default (bacs
in this case) is assumed.
<?php function enforceMinimumOrderAmount() { $paymentGatewayMinimums = [ 'paypal' => 20, 'cod' => 30, 'bacs' => 25, ]; $chosenGateway = WC()->session->get( 'chosen_payment_method' ) ?: 'bacs'; $cartTotal = WC()->cart->total; if ( isset( $paymentGatewayMinimums[$chosenGateway] ) ) { $minimumAmount = $paymentGatewayMinimums[$chosenGateway]; if ( $cartTotal < $minimumAmount ) { wc_add_notice( sprintf( esc_html__( 'The minimum order amount for %s is %s. Please add more items to your cart.', 'letsgo' ), ucfirst( $chosenGateway ), wc_price( $minimumAmount ) ), 'error' ); } } } add_action( 'woocommerce_checkout_process', 'enforceMinimumOrderAmount' );
Explanation:
- The chosen payment gateway is retrieved using
WC()->session->get( 'chosen_payment_method' )
. If no gateway is selected,bacs
is used as the default. - If the cart total is below the required minimum, an error message is added using
wc_add_notice
to prevent the checkout process.
This approach allows you to inform your customers about the required minimum amounts and ensures they cannot complete orders that do not meet the criteria. By using a default payment method, you avoid errors in cases where no gateway is selected.
Let me know if there’s anything else you’d like to adjust! 😊