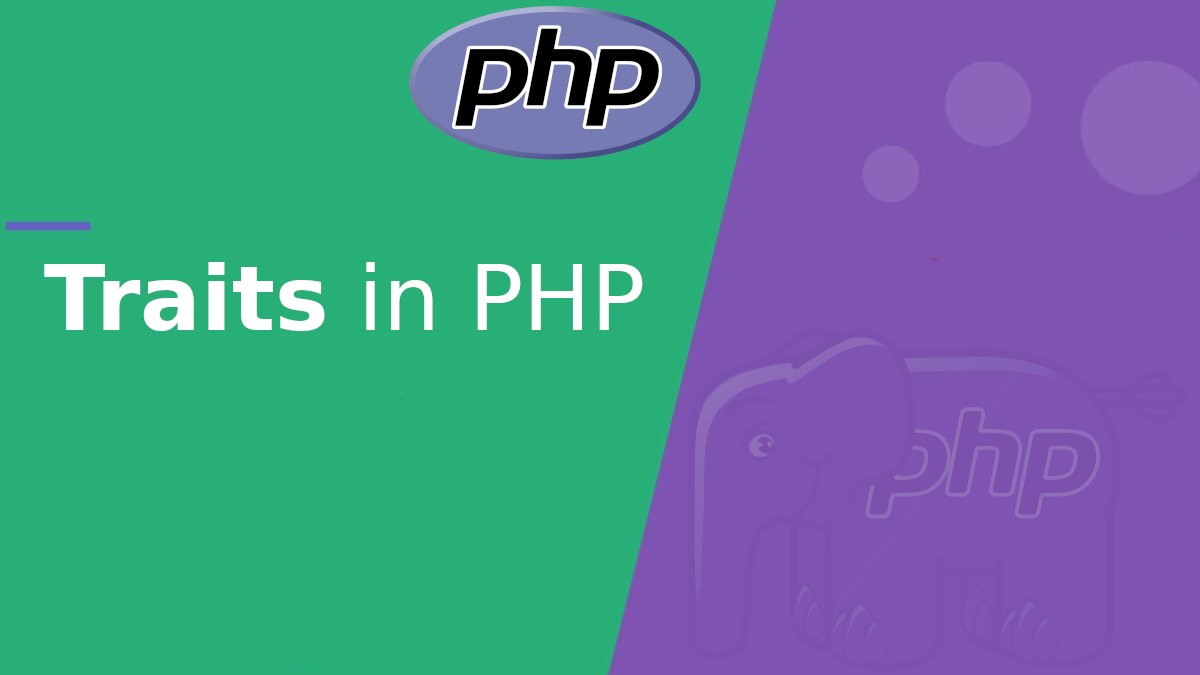
Traits in PHP
In object-oriented programming, PHP allows you to reuse code through inheritance and composition. However, when you need to combine functionalities from different classes or overcome the limitation of single inheritance, Traits are a powerful and flexible tool.
In this article, we’ll explore what Traits are, what they’re used for, and how to use them with clear examples.
What is a Trait?
A Trait is a mechanism for reusing code in PHP that allows you to include methods in a class without the need to inherit from another. Traits are not classes but blocks of code that can be reused by multiple classes.
Key Characteristics
- They enable solutions where multiple inheritance is not possible.
- They can contain methods and properties.
- They help keep code modular and clean.
What are Traits used for?
- Avoiding code duplication: Write a set of common methods in a Trait and reuse them across different classes.
- Resolving inheritance conflicts: Combine functionalities from multiple sources without inheriting from multiple classes.
- Improving code organization: Traits make it easier to break code into logical blocks.
Practical example: using Traits in PHP
The Problem: Duplicate methods
Imagine you have two classes, User
and Order
. Both need to log messages when performing certain actions, but they have no direct relationship. You could implement the log
method in both classes, but this would result in code duplication:
- The
log
method is duplicated. - If you need to modify the log format, you must do it in both classes, increasing the risk of errors.
Solution: using a Trait
We can extract the log
method into a Trait to avoid duplication:
<?php trait Logger { public function log($message) { echo "[LOG]: $message\n"; } } class User { use Logger; public function createUser($name) { $this->log("Creating user: $name"); } } class Order { use Logger; public function createOrder($id) { $this->log("Creating order: $id"); } } // Usage $user = new User(); $user->createUser("Carlos"); $order = new Order(); $order->createOrder(123);
Output
[LOG]: Creating user: Carlos [LOG]: Creating order: 123
Benefits
- The
log
method is centralized in a single location. - If you need to change its implementation, you only do so in the
Logger
Trait.
Resolving conflicts with multiple Traits
When a class uses multiple Traits with methods of the same name, PHP allows you to resolve conflicts using the insteadof
and as
keywords.
<?php trait Logger { public function log() { echo "[Logger]\n"; } } trait FileLogger { public function log() { echo "[FileLogger]\n"; } } class Report { use Logger, FileLogger { FileLogger::log insteadof Logger; // Use FileLogger's log Logger::log as logVerbose; // Alias for Logger's log } } $report = new Report(); $report->log(); // [FileLogger] $report->logVerbose(); // [Logger]
Explanation
insteadof
: Specifies which method to use when there’s a conflict.as
: Creates an alias for a method from another Trait.
Traits with properties and abstract methods
Traits can also include properties and abstract methods that the classes must implement.
<?php trait Authenticatable { private $authenticated = false; public function isAuthenticated() { return $this->authenticated; } abstract protected function login($credentials); } class Admin { use Authenticatable; protected function login($credentials) { if ($credentials === "admin123") { $this->authenticated = true; echo "User authenticated\n"; } else { echo "Invalid credentials\n"; } } } $admin = new Admin(); $admin->login("admin123"); echo $admin->isAuthenticated() ? "Yes, authenticated" : "Not authenticated";
Output
User authenticated Yes, authenticated
Advantages and disadvantages of Traits
Advantages:
- Promote code reuse.
- Facilitate the composition of functionalities.
- Solve the problem of single inheritance.
Disadvantages:
- Overuse can make the code harder to read.
- They don’t encapsulate behavior like a standalone class does.
Traits are a powerful tool in PHP to share functionality across classes efficiently and cleanly. Using them appropriately will help keep your code modular and maintainable. However, like any tool, it’s important to use them in moderation to avoid unnecessary complexity.