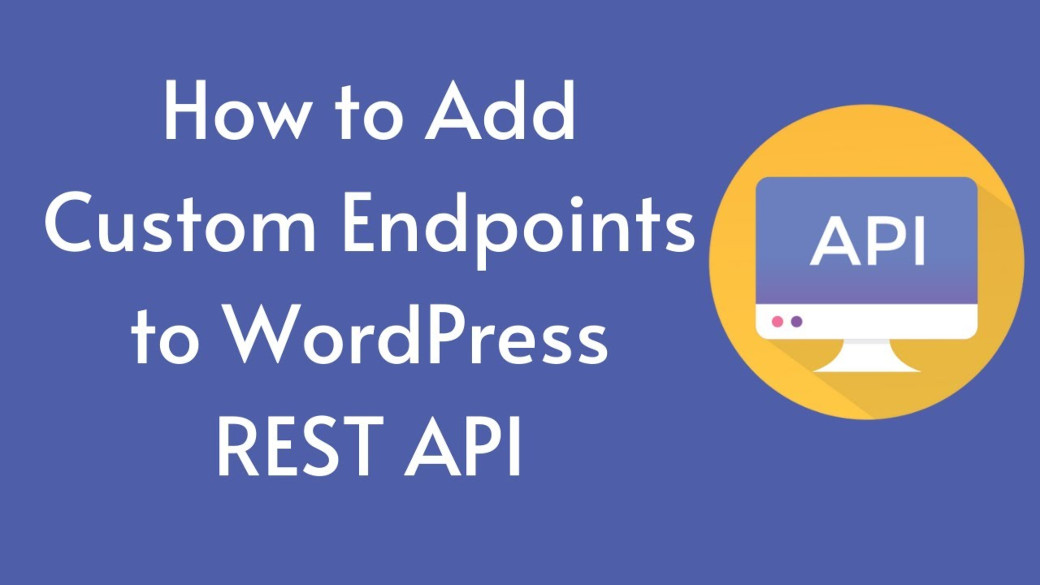
How to build a custom endpoint in WordPress
A custom endpoint in WordPress allows you to extend your site’s functionality by adding specific routes that process requests and return responses in JSON format. This is ideal for integrations and modern applications. In this article, we’ll create an endpoint that processes a parameter and returns a 200
or 400
status depending on the result.
Register the Endpoint
We use the rest_api_init
action to register the endpoint:
<?php add_action('rest_api_init', function () { register_rest_route('myApi/v1', '/processCode', [ 'methods' => 'POST', 'callback' => 'processCodeHandler', 'permission_callback' => '__return_true', // Allows public access ]); });
Create the Callback
The callback function processes the request, validates the parameters, and responds with the appropriate status.
<?php function processCodeHandler(WP_REST_Request $request) { // Retrieve the parameter sent in the request $code = $request->get_param('code'); // Validate that the parameter exists if (empty($code)) { $response = new WP_REST_Response([ 'success' => false, 'message' => 'The "code" parameter is required.', ]); $response->set_status(400); // HTTP 400 error code return $response; } // Process the code (this can be any custom logic) $result = executeCode($code); // Respond with a 200 status and the processing result $response = new WP_REST_Response([ 'success' => true, 'data' => $result, ]); $response->set_status(200); // HTTP 200 success code return $response; } function executeCode($code) { // Process the code as needed // Example: return the code in uppercase return strtoupper($code); }
In this example:
- If the
code
parameter is missing, an error message with a400
status is returned. - If the parameter is valid, the code is processed and returned with a
200
status.
Test the Endpoint
You can test the endpoint using tools like Postman, Insomnia, or curl
.
Example using curl
:
curl -X POST https://your-site.com/wp-json/myApi/v1/processCode \ -H "Content-Type: application/json" \ -d '{"code":"example text"}'
Successful Response (200)
{ "success": true, "data": "EXAMPLE TEXT" }
Error Response (400)
{ "success": false, "message": "The 'code' parameter is required." }
Add Security (Optional)
You can use a permissions callback to protect the endpoint:
'permission_callback' => function () { return current_user_can('manage_options'); // Admins only },
With this example, you have a solid foundation for creating custom endpoints in WordPress that handle both successful and error responses using the set_status()
method. Feel free to adapt it to meet your specific requirements! 😊