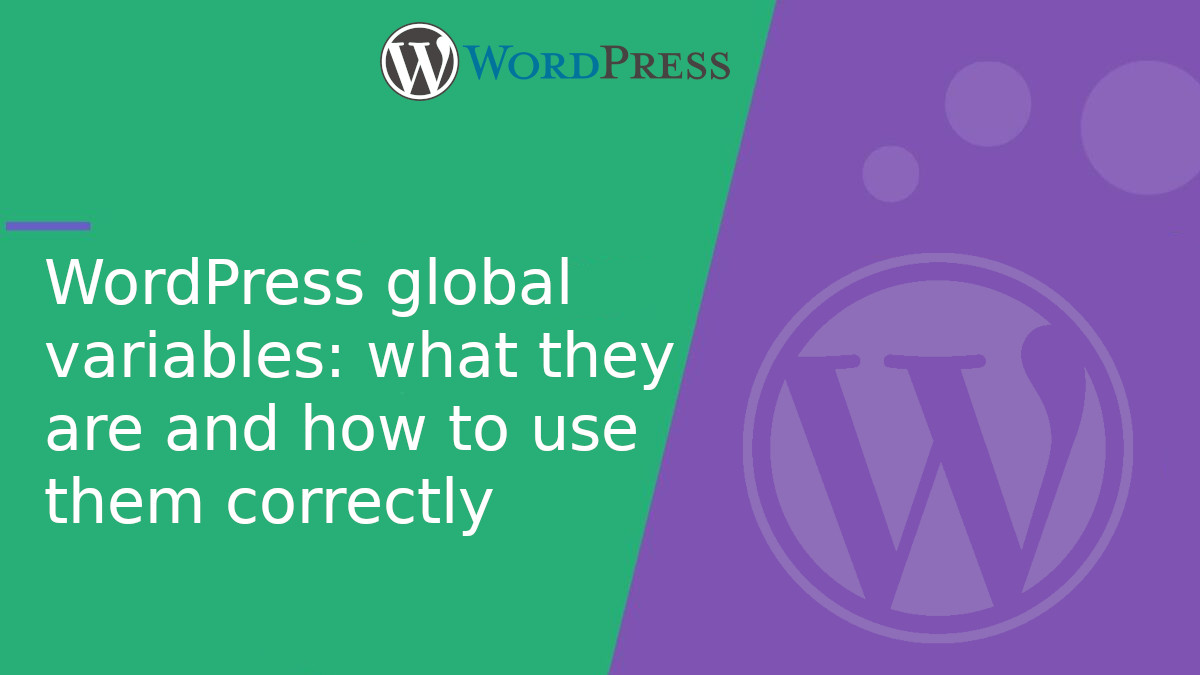
WordPress global variables: what they are and how to use them correctly
When developing plugins or themes for WordPress, it’s common to encounter various global variables that the CMS provides. These variables allow us to quickly access environment information, user data, content details, and even database query results.
In this article, we explain which WordPress global variables are the most important, what they are used for, and how you can use them properly in your development work.
What are global variables in WordPress?
In programming, a global variable is one that can be accessed from anywhere in the code. In the case of WordPress, these variables contain essential objects that are part of the system’s core.
Therefore, knowing and understanding how to use them can save you a lot of development time and help you maintain a cleaner and more efficient code structure.
Main global variables in WordPress
$wpdb
An object used to access the WordPress database. It allows you to safely perform custom SQL queries.
<?php global $wpdb; $results = $wpdb->get_results( "SELECT * FROM {$wpdb->prefix}posts WHERE post_status = 'publish'" );
$post
Contains the object of the current post
within the WordPress loop.
<?php global $post; echo $post->post_title;
$wp_query
Represents the main query that WordPress runs to load the current page.
<?php global $wp_query; echo 'Number of posts found: ' . $wp_query->found_posts;
$wp_the_query
Contains the original WordPress query before any modifications (for example, when using query_posts()
).
$wp_rewrite
An object that manages the rewriting of friendly URLs. Useful if you are registering custom rewrite rules.
<?php global $wp_rewrite; print_r( $wp_rewrite->rules );
$wp
Contains the main WordPress instance, including routes, requests, and query parameters.
$current_user
This object represents the currently logged-in user in the system.
<?php global $current_user; echo 'Current user: ' . $current_user->user_login;
You can also retrieve the current user with wp_get_current_user()
, which is a safer method.
$user_login
Contains the current user’s login name (less commonly used today, $current_user
is recommended).
$comment
Stores the current comment when inside the comments loop.
$pagenow
A variable with the name of the current PHP file being used in the WordPress admin area. Very useful for conditionals inside functions.php
.
<?php global $pagenow; if ( $pagenow === 'post-new.php' ) { // Execute something only when creating a new post }
$typenow
Contains the current post type inside the admin dashboard.
Best practices for using global variables in WordPress
- Always declare variables with global before using them.
- Avoid directly modifying their values.
- Whenever possible, use WordPress functions like
get_post()
orwp_get_current_user()
instead of relying solely on global variables.
Global variables in WordPress are powerful tools when used correctly. They allow you to access critical system information without complications. However, it’s important not to overuse them and to always follow best development practices.