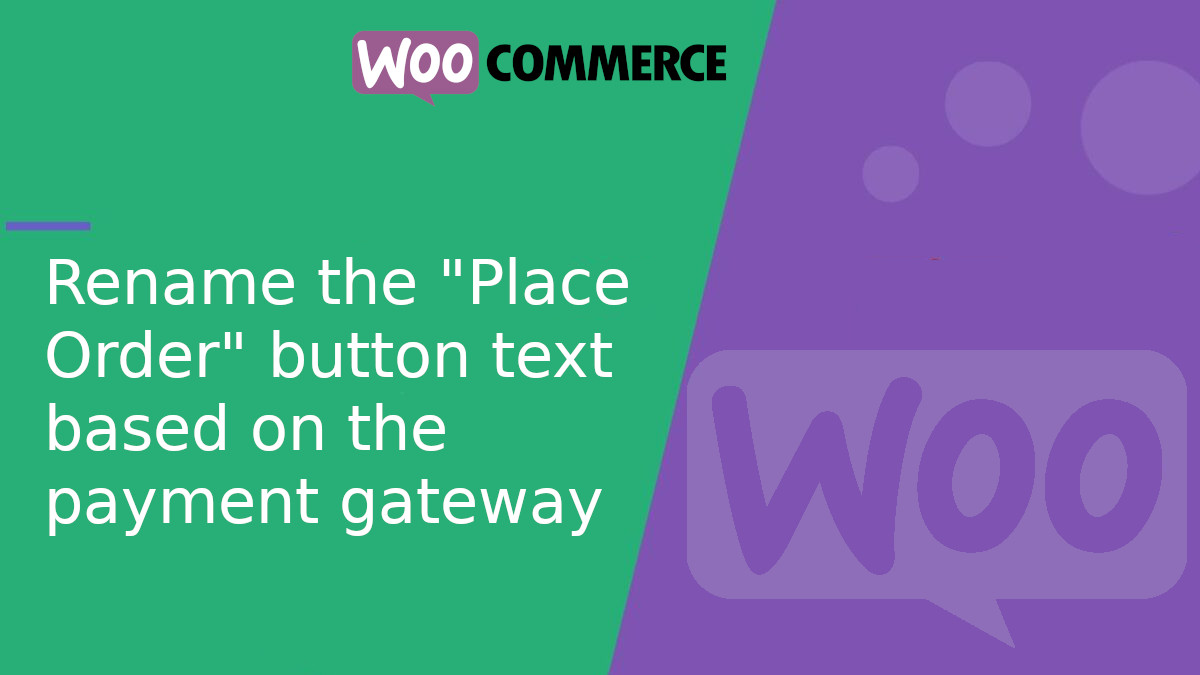
Rename the “Place Order” button text based on the payment gateway in WooCommerce
In WooCommerce, the “Place Order” button on the checkout page can be easily modified based on the selected payment gateway. This is useful when you want to customize the button text according to the payment method the user is using.
Below, we show you how to change the “Place Order” button text with a PHP snippet:
<?php function customizePlaceOrderButtonText( $buttonText, $gateway ) { // Define custom texts based on the payment gateway $customTexts = [ 'cod' => __( 'Confirm order and pay in cash', 'woocommerce' ), 'paypal' => __( 'Pay with PayPal', 'woocommerce' ), 'stripe' => __( 'Pay with credit/debit card', 'woocommerce' ), 'culqi' => __( 'Pay with Culqi', 'woocommerce' ) ]; // Check if the payment gateway has a custom text if ( isset( $customTexts[ $gateway->id ] ) ) { return $customTexts[ $gateway->id ]; } return $buttonText; } add_filter( 'woocommerce_order_button_text', 'customizePlaceOrderButtonText', 10, 2 );
Add the following code to your theme’s functions.php
file or in a custom plugin:
- We filter the button text using the
woocommerce_order_button_text
hook. - We detect the active payment gateway in the
$gateway
variable. - We create an array
$customTexts
where we define the button text for each payment method. - We check if there is a custom text for the current payment gateway and apply it.
- If the payment gateway is not listed, the original text remains unchanged.
Considerations
- You can add more payment gateways by editing the
$customTexts
array. - Make sure to use the correct identifier for the payment gateway (you can find it in WooCommerce payment settings).
- Use a child theme to prevent changes from being lost during theme updates.
With this method, you will enhance the user experience by making the checkout button more intuitive based on the selected payment gateway. We hope this snippet is useful to you!