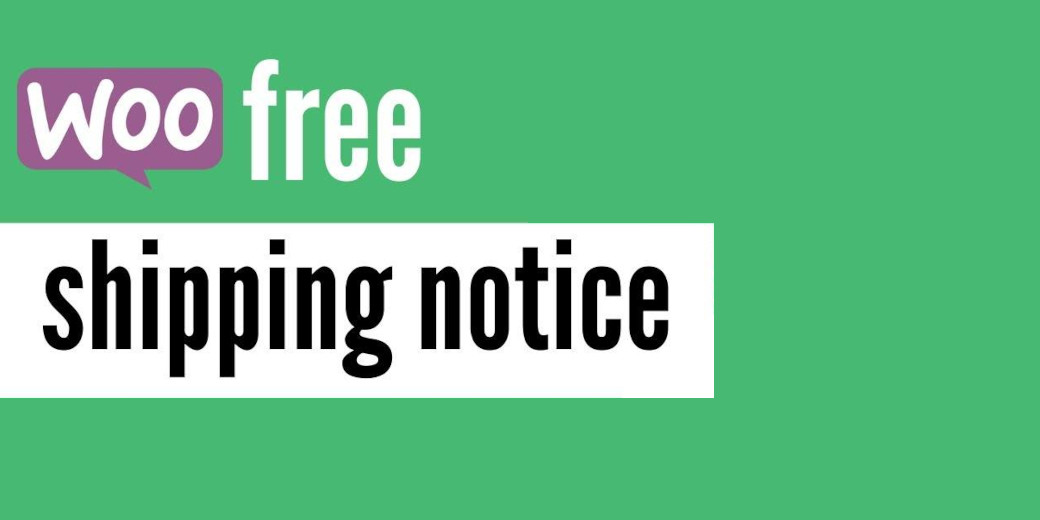
Limit amount to free shipping in WooCommerce
In an online store, offering free shipping can be an excellent strategy to increase sales. In this tutorial, you’ll learn how to display a notice to users informing them of how much they need to spend to qualify for free shipping, and how to limit the free shipping option once that condition is met.
Displaying Free Shipping Notice.
To start, we want users to know how much more they need to spend to qualify for free shipping. We’ll add a notice in the shopping cart that will inform them of the remaining amount needed.
Step 1: Open the functions.php file of your child theme or active theme.
Step 2: Add the following PHP code at the end of the file:
<?php add_action( 'woocommerce_before_cart', 'display_free_shipping_notice' ); function display_free_shipping_notice() { $min_free_shipping_amount = 100; // Define the minimum amount for free shipping $cart_total = WC()->cart->subtotal; $remaining_for_free_shipping = $min_free_shipping_amount - $cart_total; if ( $remaining_for_free_shipping > 0 ) { echo '<div class="free-shipping-notice">'; printf( 'Add %s more to your cart to qualify for free shipping!', wc_price( $remaining_for_free_shipping ) ); echo '</div>'; } }
This code will calculate how much more is needed to reach the minimum amount for free shipping and display a notice in the shopping cart if necessary.
Limiting Free Shipping.
Once the condition for free shipping is met, we want to ensure that only this shipping option is offered. To do this, we can modify the shipping options based on the situation.
Step 1: Add the following PHP code at the end of the functions.php file:
add_filter( 'woocommerce_package_rates', 'limit_free_shipping', 10, 2 ); function limit_free_shipping( $rates, $package ) { $min_free_shipping_amount = 100; // Define the minimum amount for free shipping $cart_total = WC()->cart->subtotal; if ( $cart_total >= $min_free_shipping_amount ) { // Remove all shipping methods except free shipping if cart total is greater than or equal to the minimum amount foreach ( $rates as $rate_id => $rate ) { if ( 'free_shipping' !== $rate->method_id ) { unset( $rates[ $rate_id ] ); } } } return $rates; }
This code ensures that once the cart total reaches or exceeds the minimum amount for free shipping, only that shipping option is offered by removing all others.
With these steps, you have set up a system that informs users about the remaining amount needed to qualify for free shipping and limits the shipping options once that condition is met. I hope this tutorial was helpful! If you have any questions, feel free to leave a comment!