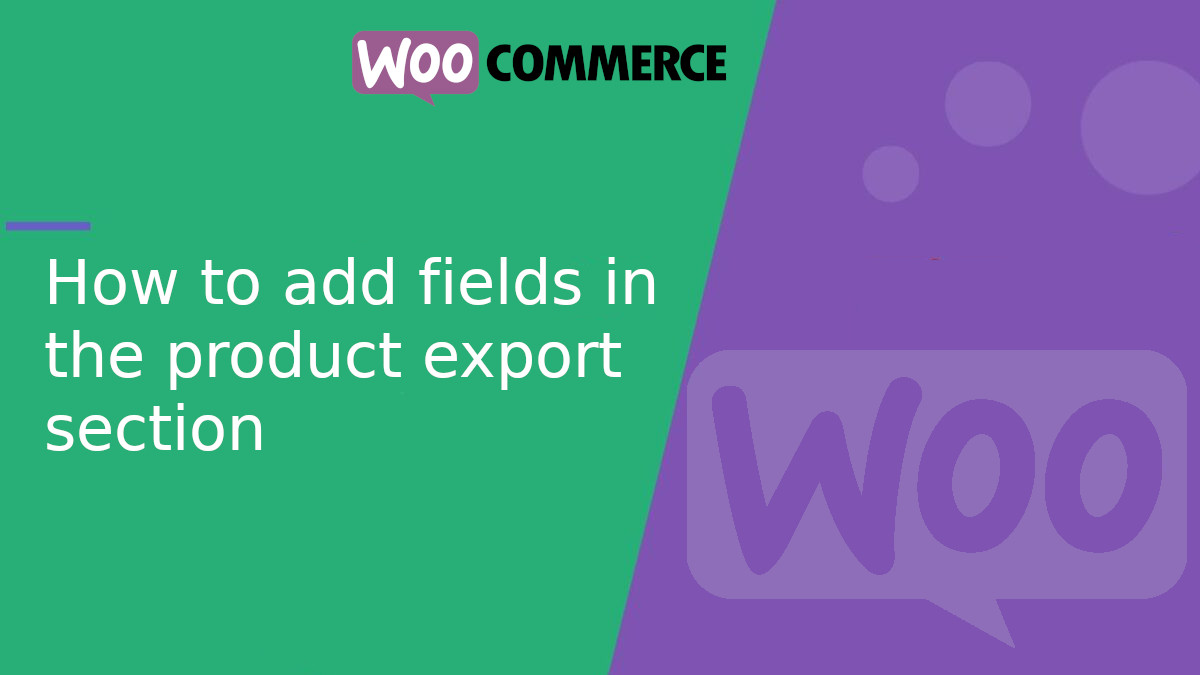
How to add fields in the WooCommerce product export section
WooCommerce allows exporting products from the WordPress admin, but by default, it does not offer the ability to add custom filters. In this article, I will show you how to add custom fields in the product export section and use them as filters to refine the export.
As an example, we will add a multi-select field to filter by product tags (product_tags
).
Adding a multi-select field in the export section
To add a custom field in the export section, we need to use the woocommerce_product_export_row
hook, which allows us to insert HTML elements within the export form.
<?php add_action( 'woocommerce_product_export_row', function() { $tags = get_terms( array( 'taxonomy' => 'product_tag', 'hide_empty' => false ) ); if ( empty( $tags ) || is_wp_error( $tags ) ) { return; } echo '<tr>'; echo '<th><label for="product_tags">' . __( 'Product Tags', 'woocommerce' ) . '</label></th>'; echo '<td>'; echo '<select id="product_tags" name="product_tags[]" class="wc-enhanced-select" multiple style="width: 100%; max-width: 300px;">'; foreach ( $tags as $tag ) { echo '<option value="' . esc_attr( $tag->slug ) . '">' . esc_html( $tag->name ) . '</option>'; } echo '</select>'; echo '</td>'; echo '</tr>'; } );
This will add a multi-select field where users can choose product tags before exporting them.
Applying a filter based on the added field
Once the field is added, we need to use the woocommerce_product_export_product_query_args
hook to modify the export query and filter the products based on the user’s selection.
<?php add_filter( 'woocommerce_product_export_product_query_args', function( $args ) { if ( isset( $_POST['form'] ) ) { parse_str( $_POST['form'], $form_data ); if ( isset( $form_data['product_tags'] ) && ! empty( $form_data['product_tags'] ) ) { $args['tax_query'][] = array( 'taxonomy' => 'product_tag', 'field' => 'slug', 'terms' => (array) $form_data['product_tags'] ); } } return $args; } );
This ensures that only the products with the selected tags are exported.
With these code snippets, you can add custom fields in the WooCommerce export section and use them to filter products before exporting them. In this example, we added a filter based on product tags, but you can apply the same methodology to other custom fields as needed.