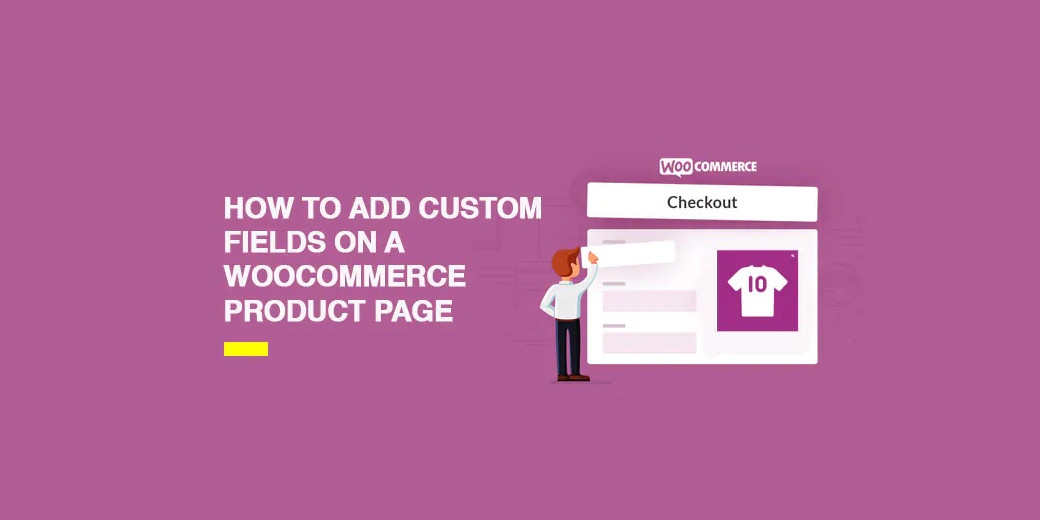
Add custom fields on WooCommerce product page
WooCommerce is an extremely flexible e-commerce platform that allows users to customize almost every aspect of their online store. One of the most common customizations is adding custom fields on the product page. These fields can be useful for collecting additional customer information, such as engravings, custom sizes, or any other data relevant to your business. In this article, we’ll explore how to add custom fields and ensure that this information is included in the final order.
Add Custom Fields to the Product Page
To add custom fields to the WooCommerce product page, we need to use WordPress filters and hooks. Here’s an example of how to add a custom field for entering a personalized message on the product page:
// Add custom field to the product form add_action('woocommerce_before_add_to_cart_button', 'add_custom_field'); function add_custom_field() { echo '<div class="custom-field"><label for="personalized_message">Personalized Message:</label><input type="text" id="personalized_message" name="personalized_message" value=""></div>'; } // Validate and save custom field when adding product to cart add_filter('woocommerce_add_cart_item_data', 'save_custom_field_to_cart', 10, 2); function save_custom_field_to_cart($cart_item_data, $product_id) { if(isset($_POST['personalized_message'])) { $cart_item_data['personalized_message'] = $_POST['personalized_message']; } return $cart_item_data; }
This code adds a text input field for the personalized message just above the “Add to Cart” button. Now, when a customer adds the product to the cart, the personalized message is saved along with the item.
Display Custom Fields in the Cart and Order
Once we’ve added the custom field to the cart, we need to make sure it’s displayed at every stage of the checkout process. To do this, we can use WooCommerce hooks to add this information to the cart and order views. Here’s how:
// Display custom field in the cart add_filter('woocommerce_get_item_data', 'display_custom_field_in_cart', 10, 2); function display_custom_field_in_cart($cart_data, $cart_item) { $custom_items = array(); if(!empty($cart_item['personalized_message'])) { $custom_items[] = array( 'name' => __('Personalized Message', 'woocommerce'), 'value' => $cart_item['personalized_message'] ); } return !empty($custom_items) ? array_merge($cart_data, $custom_items) : $cart_data; } // Display custom field in the order add_action('woocommerce_checkout_create_order_line_item', 'display_custom_field_in_order', 10, 4); function display_custom_field_in_order($item, $cart_item_key, $values, $order) { if(!empty($values['personalized_message'])) { $item->add_meta_data(__('Personalized Message', 'woocommerce'), $values['personalized_message']); } }
With this code, the custom field will be displayed both on the cart page and the order page, allowing the customer to review their personalized message before completing the purchase.
Adding custom fields on the WooCommerce product page and ensuring that these fields are included in the final order can enhance the customer experience and provide additional information to meet their needs. With the right WordPress and WooCommerce filters and hooks, this process can be achieved easily and effectively, tailored to the specific needs of your online store. I hope this article has been helpful in understanding how to implement this functionality on your WooCommerce website.