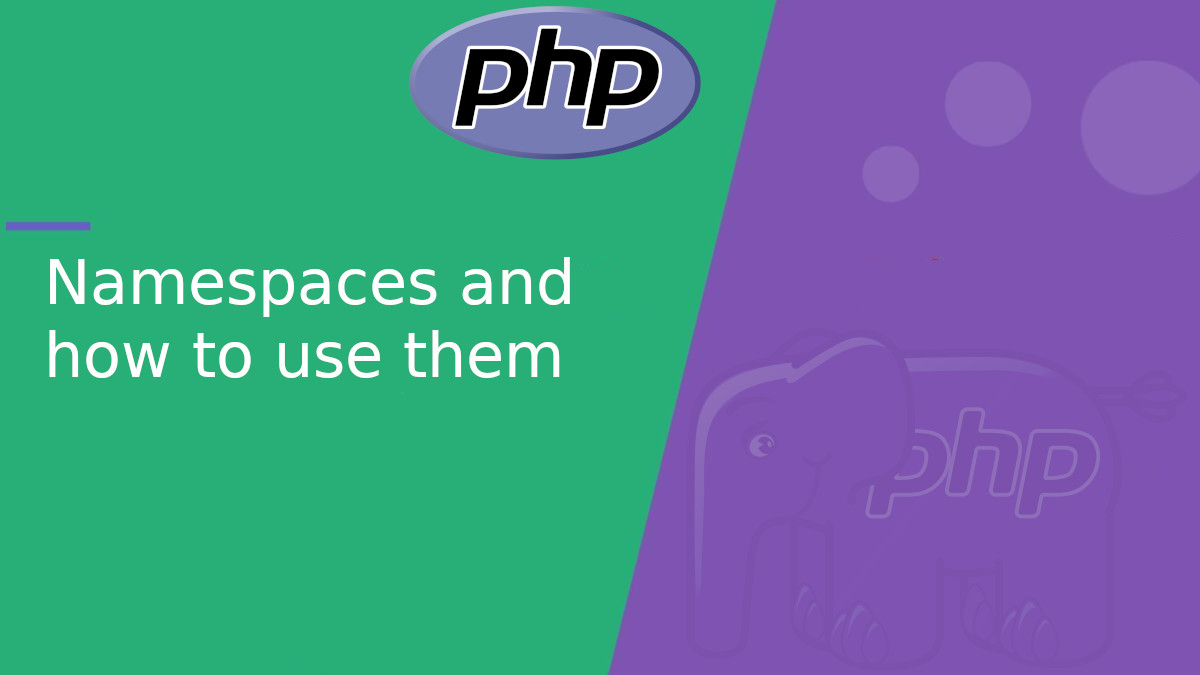
Understanding PHP Namespaces
Namespaces in PHP allow organizing code and avoiding collisions between class, function, or constant names when working on large projects or integrating multiple libraries.
What is a Namespace?
A namespace is a container that groups classes, functions, and constants under a specific name. This is useful to prevent conflicts when different parts of the code have similar names.
For example, if two different libraries define a class called Database
, they might conflict if namespaces are not used.
Defining a Namespace
To declare a namespace in PHP, use the namespace
keyword at the beginning of the file. Let’s see an example:
<?php namespace MyProject; // Defining a namespace called MyProject class Database { public function connect() { return "Connecting to the database"; } }
Now, this Database
class belongs to the MyProject
namespace and will not conflict with other classes named Database
in different parts of the project.
Using a Namespace
To use a class within a namespace, reference it with the namespace name:
<?php require 'MyProject/Database.php'; $db = new MyProject\Database(); echo $db->connect();
Here, MyProject\Database
is used to correctly access the Database
class defined within the MyProject
namespace.
Alias with use
To avoid writing the full namespace every time, you can use the use
keyword to assign an alias:
<?php use MyProject\Database; $db = new Database(); echo $db->connect();
This makes the code cleaner and easier to read.
Nested Namespaces
Namespaces can be structured into sublevels to better organize the code:
<?php namespace MyProject\Models; class User { public function getName() { return "John Doe"; } }
To use this class from another file:
<?php use MyProject\Models\User; $user = new User(); echo $user->getName();
Using Namespaces in functions
Namespaces can also contain functions, helping to avoid name conflicts between similar functions in different files.
<?php namespace MyProject\Utils; function greet() { return "Hello from MyProject!"; }
To use this function in another file:
<?php use function MyProject\Utils\greet; echo greet();
Using Namespaces and Traits
Traits in PHP can be combined with namespaces to improve code organization.
<?php namespace MyProject\Traits; trait Logger { public function log($message) { echo "[LOG]: " . $message; } }
To use this Trait in a class:
<?php namespace MyProject\Models; use MyProject\Traits\Logger; class Product { use Logger; } $product = new Product(); $product->log("Product created");
Namespaces in PHP are essential for organizing code and avoiding name collisions. Using them correctly improves project maintainability and scalability.
It is advisable to define clear namespaces and use use
to facilitate their use in the code.