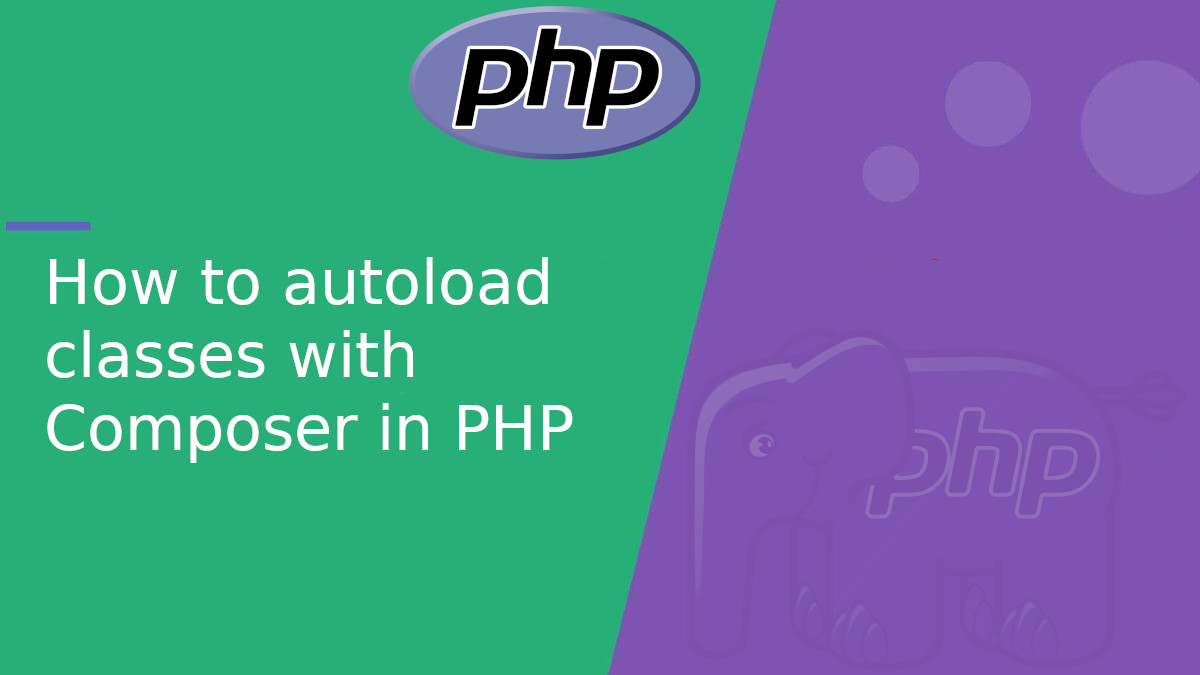
How to autoload classes with Composer in PHP
Composer is an essential tool for dependency management in modern PHP projects. One of its most powerful features is autoloading, which lets you automatically load classes and helper files without including them manually. In this guide, we’ll configure Composer to autoload both classes and simple PHP files using namespaces.
Define the initial project structure
Before starting, let’s define the basic file structure of the project. This will help you organize and visualize how the classes and helpers are integrated.
src/
will contain both namespaced classes and helper files.composer.json
will define the autoloading rules.index.php
will be the entry point to test the code.
Create a PHP project
- Create a new directory for your project and navigate to it:
- Initialize Composer inside the directory:
Follow the prompts to configure the
composer.json
file. You can accept the default values for this tutorial.
Configure autoloading in composer.json
Edit the composer.json
file to set up autoload rules for classes and helpers:
App\\
defines the main namespace.src/
is the directory where your classes will be stored.files
allows you to include simple PHP files like helpers.
Create the required files
- Create the
src/
directory: - Inside
src/
, create the fileMyClass.php
: - Also, create the
helpers.php
file to define common functions:
Generate the autoload file
Run the following command to let Composer generate the autoload file:
After this step, your project structure will look like this:
Use classes and helpers
Create the index.php
file at the root of your project with the following content:
Run the file from the terminal:
You should see the following output:
Hello, World! HELLO WORLD
Composer greatly simplifies the process of autoloading classes and helpers in PHP. Using namespaces, PSR-4 autoloading, and included files, you can keep your project organized and scalable. Define your file structure well, experiment with this configuration, and enjoy the benefits of efficient code management.