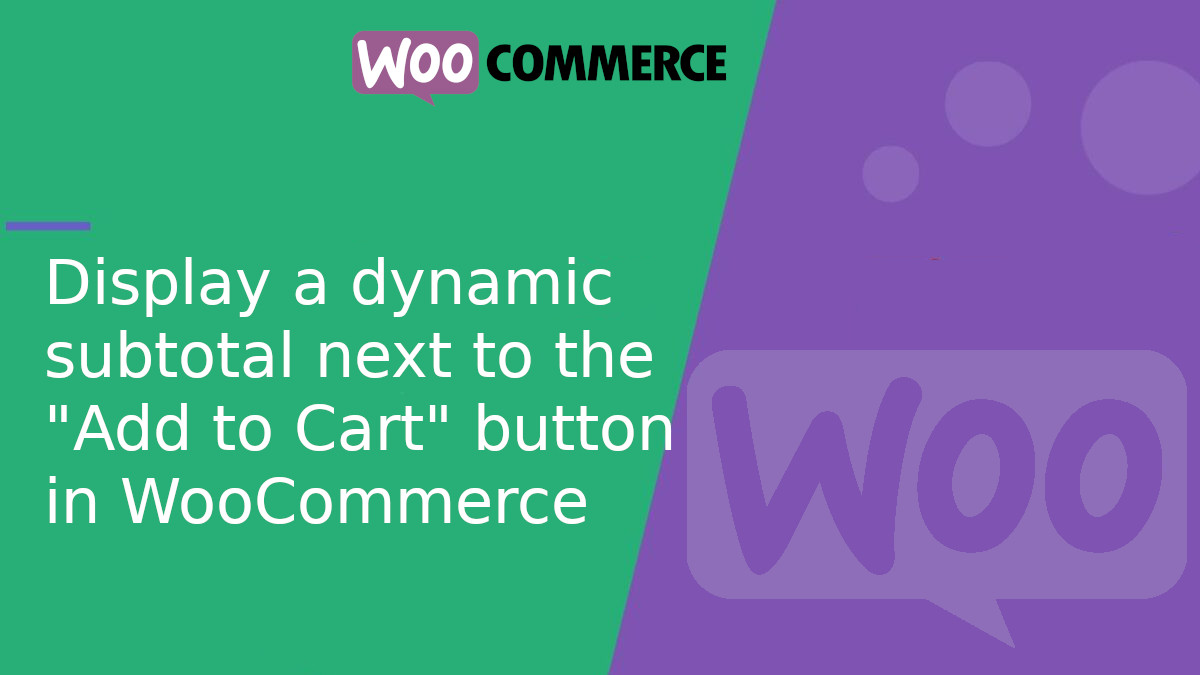
Display a dynamic subtotal next to the “Add to Cart” button in WooCommerce Products
Would you like to enhance your customers’ experience by showing a dynamic subtotal on the WooCommerce product page, right next to the “Add to cart” button? In this article, we’ll show you how to do it easily, supporting both simple and variable products, using a bit of PHP and JavaScript.
What will we achieve?
We’ll add a small block below the quantity selector that displays the subtotal (unit price × selected quantity) and automatically updates as the user changes the quantity.
Add the HTML to display the subtotal
We’ll use a WooCommerce hook to insert a container that shows the subtotal. Add this snippet to your child theme’s functions.php
file or to a custom plugin:
<?php add_action( 'woocommerce_after_add_to_cart_quantity', 'addSubtotalDisplay' ); function addSubtotalDisplay() { echo '<p id="productSubtotal" style="margin-top:10px; font-weight:bold;"></p>'; }
Add the JavaScript to update the subtotal
Now we’ll add the script that calculates and displays the subtotal each time the customer changes the quantity. WooCommerce already prints the unit price on the page, so we can easily capture it.
<?php add_action( 'wp_footer', 'addSubtotalScript' ); function addSubtotalScript() { if ( ! is_product() ) { return; } ?> <script> (function($){ function updateSubtotalDisplay() { var quantityInput = $('form.cart').find('input.qty'); var priceElement = $('.woocommerce-Price-amount').first(); var subtotalElement = $('#productSubtotal'); if ( priceElement.length === 0 || quantityInput.length === 0 || subtotalElement.length === 0 ) { return; } var priceText = priceElement.text().replace(/[^0-9.,]/g, '').replace(',', '.'); var unitPrice = parseFloat(priceText); var quantity = parseInt(quantityInput.val()); var subtotal = (unitPrice * quantity).toFixed(2); var currencySymbol = priceElement.text().replace(/[0-9.,]/g, '').trim(); subtotalElement.text('Subtotal: ' + currencySymbol + subtotal); } $(document).ready(function(){ updateSubtotalDisplay(); $('form.cart').on('change input', 'input.qty', function(){ updateSubtotalDisplay(); }); $('form.variations_form').on('woocommerce_variation_has_changed', function(){ setTimeout(updateSubtotalDisplay, 100); // Wait for WooCommerce to update the price }); }); })(jQuery); </script> <?php }
How Does It Work?
- The HTML is inserted just below the quantity selector.
- The script grabs the first visible price (
.woocommerce-Price-amount
), extracts the numeric value, and multiplies it by the selected quantity. - For variable products, WooCommerce updates the price via AJAX. That’s why we use the
woocommerce_variation_has_changed
event to recalculate the subtotal when a variation is selected.
With just a few lines of PHP and JavaScript, you can significantly improve the user experience in your WooCommerce store. Displaying a dynamic subtotal is a simple but powerful way to bring clarity and trust to the buying process.
Would you like more tips like this? Let me know and we’ll prepare more practical WooCommerce guides for your store!