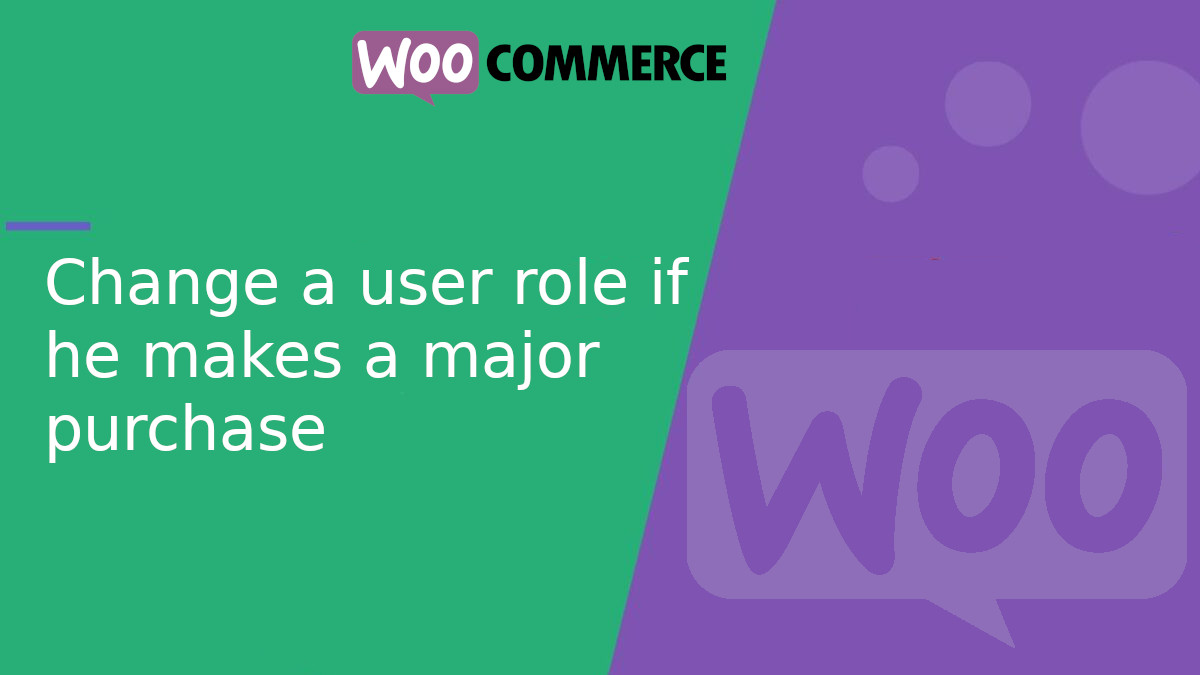
Change a user role if he makes a major purchase
In this article, you will learn how to automatically change a user’s role in WooCommerce if they make a purchase above a specific amount. For this example, we will create a new role called “Vip User” and assign this role to users who meet the criteria.
Create the “Vip User” role
Before changing users’ roles, we must ensure that the “Vip User” role exists. To do this, we can add the following code to our theme’s functions.php
file or a custom plugin:
<?php function create_vip_user_role() { add_role( 'vip_user', 'Vip User', [ 'read' => true, 'edit_posts' => false, 'delete_posts' => false, ]); } add_action( 'init', 'create_vip_user_role' );
This code defines the “Vip User” role with basic read permissions.
Change user role after a purchase
To assign the “Vip User” role to a buyer who exceeds a specific amount, we can use the following snippet:
<?php function change_user_role_on_high_purchase( $order_id ) { // Get the order $order = wc_get_order( $order_id ); if ( ! $order ) { return; } // Get the user $user_id = $order->get_user_id(); if ( ! $user_id ) { return; } // Define the minimum amount to become VIP $min_vip_amount = 100; // Change this to the desired amount // Get the order total $order_total = $order->get_total(); // Check if the total exceeds the minimum if ( $order_total >= $min_vip_amount ) { $user = new WP_User( $user_id ); // Assign the new role and remove the previous one $user->set_role( 'vip_user' ); } } add_action( 'woocommerce_thankyou', 'change_user_role_on_high_purchase' );
- We get the order object with
wc_get_order( $order_id )
. - Extract the
user_id
of the buyer. - Define a minimum purchase amount to be considered a “Vip User”.
- Compare the purchase total with the minimum amount.
- If the amount is equal to or greater than the threshold, assign the “Vip User” role to the user.
Testing the Code
To verify that the role change works correctly:
- Make a purchase with a test user.
- Ensure that the purchase total exceeds the defined amount.
- Check under Users > All Users in WordPress to see if the role has changed to “Vip User”.
With this method, you can easily implement a VIP membership system based on purchases in your WooCommerce store.