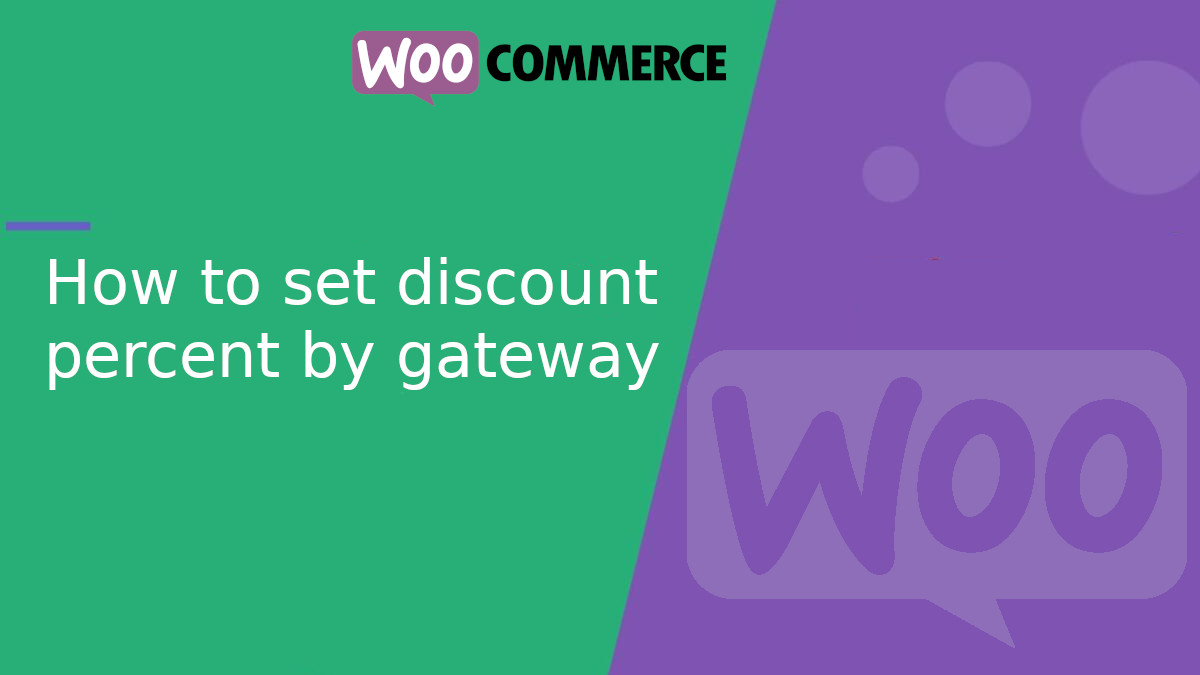
How set discount percent by gateway in WooCommerce
In WooCommerce, you can encourage the use of specific payment gateways by offering automatic discounts depending on the method selected by the customer. This article will show you how to implement this feature using the WooCommerce hooks.
WooCommerce stores the selected payment method in the customer’s session during the checkout process. This information is accessible via WC()->session->get( 'chosen_payment_method' )
. The value returned corresponds to the payment gateway identifier (e.g., paypal, cod, etc.), and this will be used to determine if a discount should be applied and how much.
This built-in functionality makes it easy to retrieve the payment method without needing additional logic to capture it manually.
Define discounts and apply them to the cart
Now that we know how to get the selected payment method, we’ll use the woocommerce_cart_calculate_fees
hook to define and apply discounts based on the payment gateway.
<?php add_action( 'woocommerce_cart_calculate_fees', 'applyDiscountBasedOnPaymentMethod' ); function applyDiscountBasedOnPaymentMethod( $cart ) { // Avoid executing in the admin area. if ( is_admin() && !defined( 'DOING_AJAX' ) ) { return; } // Get the selected payment method from the WooCommerce session. $chosenGateway = WC()->session->get( 'chosen_payment_method' ); // Check if a payment method is selected. if ( empty( $chosenGateway ) ) { return; } // Define discounts for each payment method. $discounts = array( 'cod' => 10, // 10% discount for Cash on Delivery. 'paypal' => 5, // 5% discount for PayPal. 'stripe' => 7, // 7% discount for Stripe. 'bank_transfer' => 3, // 3% discount for Bank Transfer. ); // Apply the discount if the payment method has a defined discount. if ( array_key_exists( $chosenGateway, $discounts ) ) { $discountPercentage = $discounts[ $chosenGateway ]; $cartTotal = $cart->get_subtotal(); $discountAmount = $cartTotal * ( $discountPercentage / 100 ); // Add the discount to the cart as a negative fee. $cart->add_fee( sprintf( __( 'Discount %s (%s%%)', 'text-domain' ), ucfirst( $chosenGateway ), $discountPercentage ), -$discountAmount ); } }
Verify the functionality
- Add the code to your active theme’s
functions.php
file or use a custom plugin to insert the functionality. - Configure the payment gateways in WooCommerce.
- Test the checkout process to ensure the discount is applied based on the selected payment method.
WooCommerce makes it easy to implement discounts based on the selected payment gateway using its session functionality. Leveraging this feature can improve the customer experience and optimize costs associated with specific payment methods.