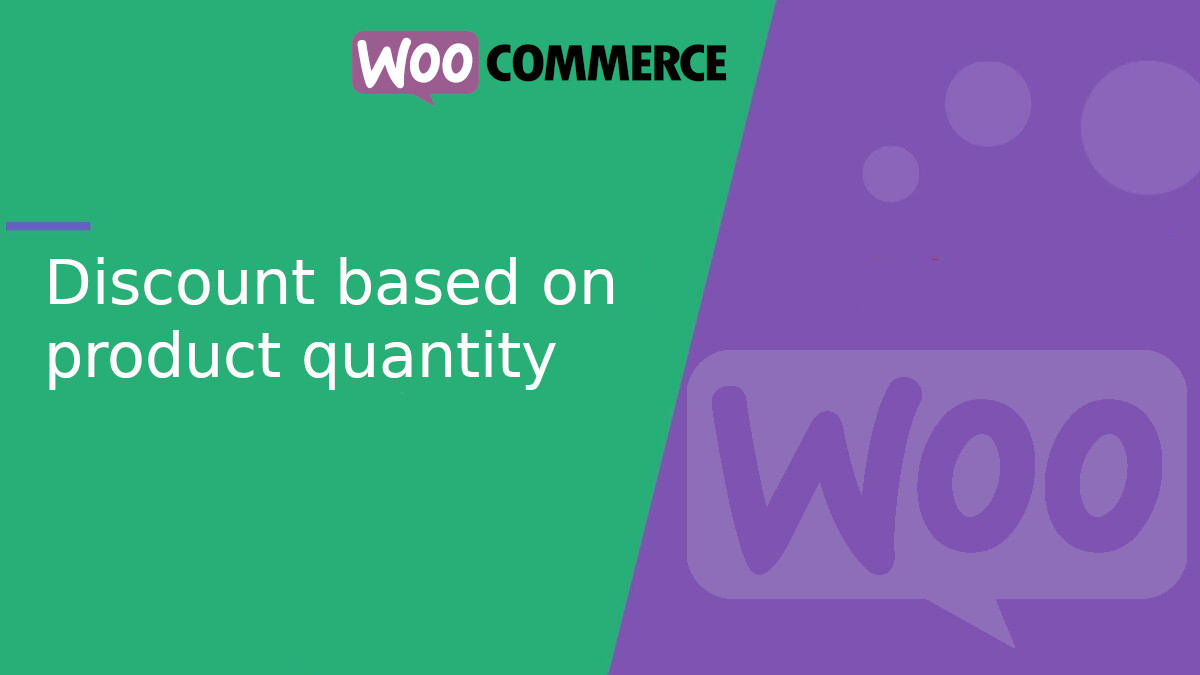
How to create discounts based on product quantity in WooCommerce
In WooCommerce, you can implement advanced discount rules based on the number of products in the cart. While there are plugins to achieve this, creating custom code provides greater flexibility and control. In this article, you’ll learn how to apply automatic discounts using PHP.
Use the woocommerce_cart_calculate_fees
hook
The woocommerce_cart_calculate_fees
hook allows you to add additional charges or discounts to the cart. We’ll use this hook to implement the quantity-based discount.
Basic example: discount for 10 or more products
<?php add_action( 'woocommerce_cart_calculate_fees', 'applyQuantityBasedDiscount' ); function applyQuantityBasedDiscount( $cart ) { // Ensure it doesn't run in the backend. if ( is_admin() && !defined( 'DOING_AJAX' ) ) { return; } // Minimum quantity to apply the discount. $minQuantity = 10; // Discount percentage. $discountPercentage = 10; // Calculate the total number of items in the cart. $totalQuantity = $cart->get_cart_contents_count(); if ( $totalQuantity >= $minQuantity ) { // Calculate the discount. $discount = $cart->subtotal * ( $discountPercentage / 100 ); // Apply the discount as a negative fee. $cart->add_fee( __( 'Quantity Discount', 'your-text-domain' ), -$discount ); } }
Tiered Quantity Discounts
You can offer different discounts based on the number of products in the cart.
Example: progressive discounts
<?php add_action( 'woocommerce_cart_calculate_fees', 'applyTieredQuantityDiscount' ); function applyTieredQuantityDiscount( $cart ) { if ( is_admin() && !defined( 'DOING_AJAX' ) ) { return; } $totalQuantity = $cart->get_cart_contents_count(); $discount = 0; // Define the discount tiers. if ( $totalQuantity >= 20 ) { $discount = $cart->subtotal * 0.20; // 20% discount for 20 or more products. } elseif ( $totalQuantity >= 10 ) { $discount = $cart->subtotal * 0.10; // 10% discount for 10-19 products. } if ( $discount > 0 ) { $cart->add_fee( __( 'Tiered Discount', 'your-text-domain' ), -$discount ); } }
Discounts for a specific product
If you want to apply discounts only when multiple units of a specific product are in the cart, you can check the cart items.
Example: discount for buying 5 or more of a specific product
<?php add_action( 'woocommerce_cart_calculate_fees', 'applyProductSpecificDiscount' ); function applyProductSpecificDiscount( $cart ) { if ( is_admin() && !defined( 'DOING_AJAX' ) ) { return; } // Target product ID. $targetProductId = 123; // Replace this with your product ID. $minQuantity = 5; // Minimum quantity for the discount. $discountPercentage = 15; // Discount percentage. foreach ( $cart->get_cart() as $cartItem ) { if ( $cartItem['product_id'] == $targetProductId && $cartItem['quantity'] >= $minQuantity ) { // Calculate the discount for the specific product. $discount = ( $cartItem['line_total'] * $discountPercentage ) / 100; // Apply the discount. $cart->add_fee( __( 'Product Discount', 'your-text-domain' ), -$discount ); break; } } }
Creating quantity-based discounts in WooCommerce is an excellent way to encourage larger purchases and boost sales. With the examples above, you can customize discounts to meet your store’s needs.
If you have any questions or need more examples, feel free to ask! 😊