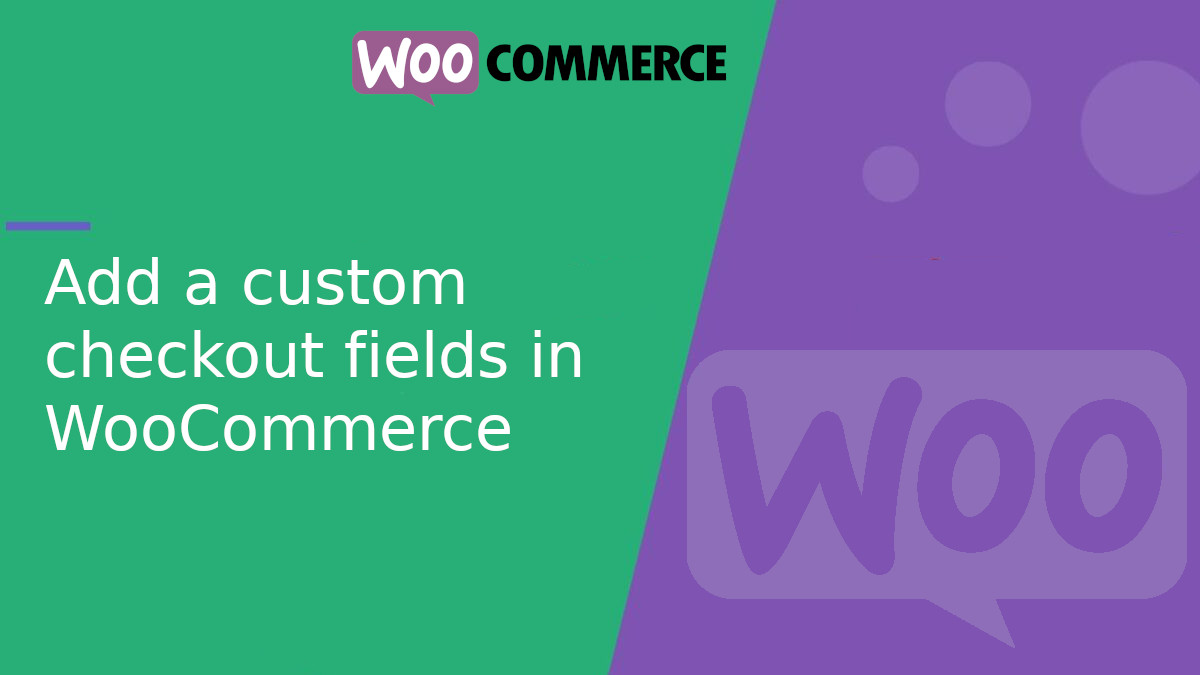
Add a custom field to WooCommerce Checkout
WooCommerce is an extremely flexible platform that allows you to customize various aspects of your online store. One of these customizations includes adding custom fields to the checkout. This tutorial will guide you step-by-step on how to add a custom field to the WooCommerce checkout using PHP.
Add the custom field to the checkout form
To add a new field to the checkout form, we’ll use the woocommerce_checkout_fields
filter. This filter allows us to modify the default checkout fields or add new ones.
<?php add_filter('woocommerce_checkout_fields', 'add_custom_checkout_field'); function add_custom_checkout_field($fields) { $fields['billing']['billing_custom_field'] = array( 'type' => 'text', 'label' => __('Custom Field', 'your-text-domain'), 'placeholder' => __('Enter your value', 'your-text-domain'), 'required' => true, 'class' => array('form-row-wide'), 'priority' => 25, ); return $fields; }
In this example, we added a field calle billing_custom_field
to the billing fields group (billing
). The field is a required text input.
Validate the custom field
To ensure the field is properly completed, we can add custom validation using the woocommerce_checkout_process
hook.
<?php add_action('woocommerce_checkout_process', 'validate_custom_checkout_field'); function validate_custom_checkout_field() { if (empty($_POST['billing_custom_field'])) { wc_add_notice(__('Please fill in the custom field.', 'your-text-domain'), 'error'); } }
This function checks if the billing_custom_field
is empty and displays an error message if it is not filled in.
Save the custom field value
After validating the field, we need to save its value in the order. For this, we’ll use the woocommerce_checkout_update_order_meta
hook.
<?php add_action('woocommerce_checkout_update_order_meta', 'save_custom_checkout_field'); function save_custom_checkout_field($order_id) { if (!empty($_POST['billing_custom_field'])) { update_post_meta($order_id, '_billing_custom_field', sanitize_text_field($_POST['billing_custom_field'])); } }
Here, we are storing the custom field value as an order meta field.
Display the custom field in the admin panel
To ensure that administrators can see the value entered by the customer, we’ll use the woocommerce_admin_order_data_after_billing_address
hook.
<?php add_action('woocommerce_admin_order_data_after_billing_address', 'display_custom_checkout_field_admin', 10, 1); function display_custom_checkout_field_admin($order) { $custom_field_value = get_post_meta($order->get_id(), '_billing_custom_field', true); if (!empty($custom_field_value)) { echo '<p><strong>' . __('Custom Field', 'your-text-domain') . ':</strong> ' . esc_html($custom_field_value) . '</p>'; } }
With this code, the custom field value will be displayed in the billing section of the order admin panel.
Display the custom field in order notifications
If you want to include this field in emails or the customer’s order summary, you can use the woocommerce_email_order_meta
hook.
<?php add_filter('woocommerce_email_order_meta_fields', 'add_custom_checkout_field_to_email', 10, 3); function add_custom_checkout_field_to_email($fields, $sent_to_admin, $order) { $custom_field_value = get_post_meta($order->get_id(), '_billing_custom_field', true); if (!empty($custom_field_value)) { $fields['custom_field'] = array( 'label' => __('Custom Field', 'your-text-domain'), 'value' => $custom_field_value, ); } return $fields; }
With this code, the field value will appear in emails related to the order.
Customizing WooCommerce checkout is an excellent way to tailor your store to your customers’ needs. By following the steps above, you can add and manage a custom field using PHP. This example is fully functional, but you can always adapt it to add more fields or implement more complex validations.