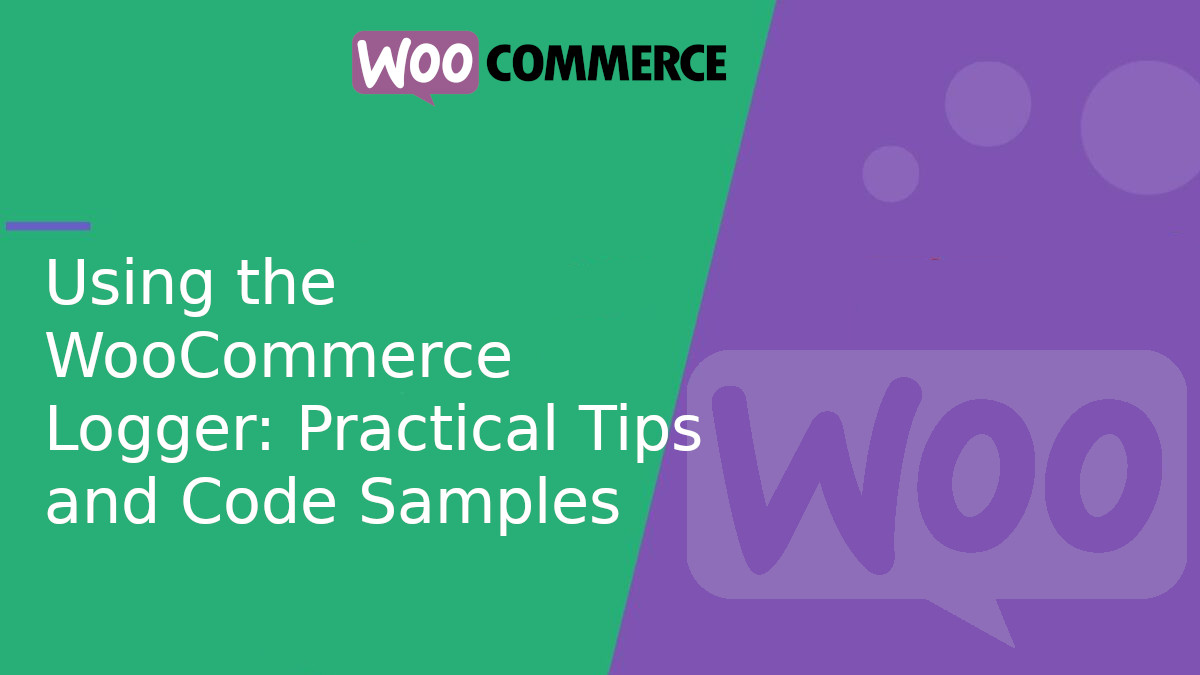
Using the WooCommerce Logger: Practical Tips and Code Samples
WooCommerce provides a powerful logging system that helps you track events, errors, API responses, or any relevant data for debugging purposes. This system is based on the WC_Logger
class and is very flexible. In this article, you’ll learn how to use it in your custom developments.
Why Use the WooCommerce Logging System?
The WooCommerce logging system allows you to:
- Log debug messages or errors.
- Store responses from external services (e.g., payment gateways).
- View logs directly from the WordPress admin dashboard.
- Keep logs organized and separated by source.
Log File Location
Logs are stored in:
wp-content/uploads/wc-logs/
Starting from WooCommerce 3.4, logs are handled by the WC_Log_Handler_File
class and can be viewed from the admin panel at: WooCommerce > Status > Logs
How to Write Messages to a Custom Log
You can use the WC_Logger
class to write to logs. Here’s a basic example:
<?php function myPluginLogExample() { $logger = wc_get_logger(); $context = [ 'source' => 'my-plugin' ]; $logger->info( 'This is an info message.', $context ); $logger->warning( 'This is a warning message.', $context ); $logger->error( 'This is an error message.', $context ); }
This will create a log file named:
log-my-plugin-[date].log
How to View Logs
From the WordPress admin panel:
- Go to
WooCommerce > Status > Logs
. - Select the file named
my-plugin
(or the source you used). - View the logged entries.
Practical Example: Logging Errors from an API Call
Let’s say you’re making a request to an external API and want to log both the request and the response:
<?php function callExternalApiAndLog() { $logger = wc_get_logger(); $logContext = [ 'source' => 'external-api' ]; $requestData = [ 'name' => 'John Doe', 'email' => 'john@example.com' ]; $logger->info( 'Sending data to API: ' . json_encode( $requestData ), $logContext ); $response = wp_remote_post( 'https://api.external.com/endpoint', [ 'method' => 'POST', 'body' => json_encode( $requestData ), 'headers' => [ 'Content-Type' => 'application/json' ] ] ); if ( is_wp_error( $response ) ) { $logger->error( 'Request error: ' . $response->get_error_message(), $logContext ); return; } $responseBody = wp_remote_retrieve_body( $response ); $logger->info( 'API response: ' . $responseBody, $logContext ); }
How to Add Conditional Logging (Debug Mode)
To avoid logging too much in production, you can conditionally log messages only in debug mode:
<?php function conditionalLogExample( $message ) { if ( defined( 'WP_DEBUG' ) && WP_DEBUG ) { $logger = wc_get_logger(); $context = [ 'source' => 'debug-mode' ]; $logger->debug( $message, $context ); } }
The WooCommerce logging system is a powerful tool that helps you understand what’s happening behind the scenes in your plugins. Whether you’re integrating with external services or simply want to monitor plugin behavior, implementing logs will help you detect issues and improve your development quality.