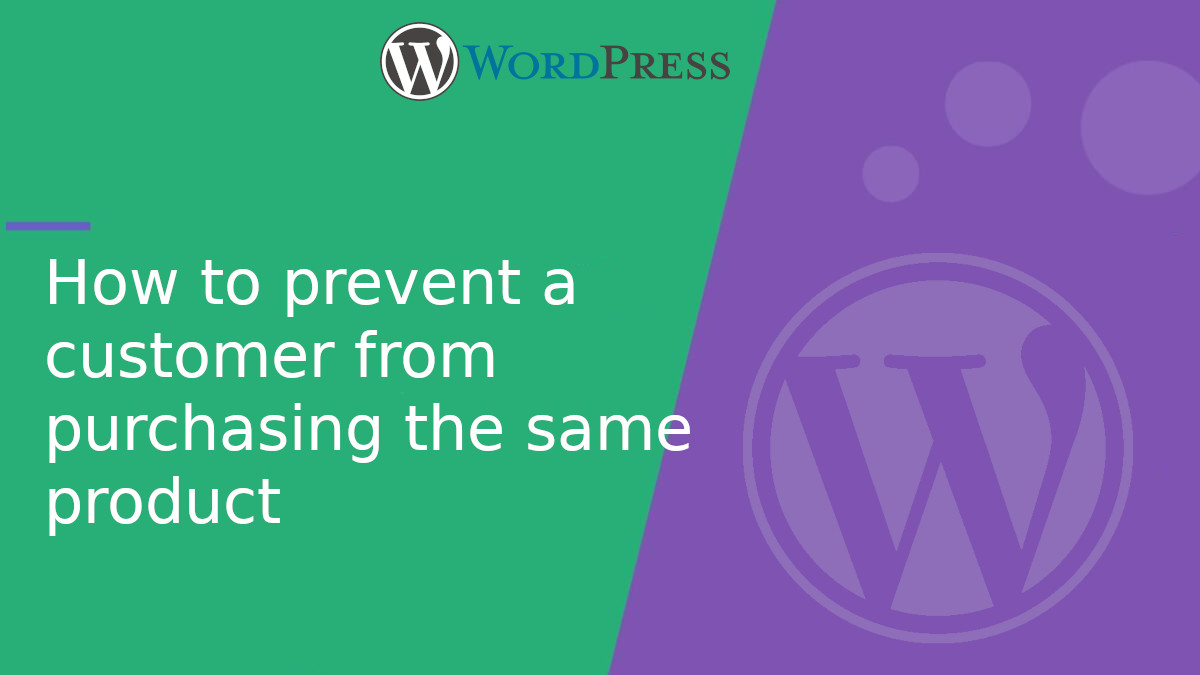
How to prevent a customer from purchasing the same product more than once in WooCommerce
In certain WooCommerce stores—especially those selling unique items such as licenses, memberships, or digital products—it may be necessary to prevent a customer from purchasing the same product more than once. In this article, we’ll show you how to do it using simple code snippets and best practices.
Important Requirement: In order to restrict purchases per customer, users must be logged in. If you haven’t enforced login yet, here’s how to do it.
Force login before allowing purchases
By default, WooCommerce allows guest checkout. To disable this:
- Go to: WooCommerce > Settings > Accounts & Privacy
- And uncheck the following options:
- Allow customers to place orders without an account.
- Allow customers to create an account during checkout (optional, depending on your flow).
Alternatively, you can force login using the snippet below:
<?php add_action( 'template_redirect', function() { if ( ! is_user_logged_in() && is_product() ) { wp_redirect( wp_login_url( get_permalink() ) ); exit; } } );
This snippet redirects visitors to the login page if they try to access a product without being logged in.
Hide the “add to cart” button if already purchased
A simple and visual solution is to hide the “Add to cart” button for products already purchased by the logged-in user.
<?php add_filter( 'woocommerce_is_purchasable', 'hideAddToCartIfPurchased', 10, 2 ); function hideAddToCartIfPurchased( $purchasable, $product ) { if ( is_user_logged_in() && hasUserPurchasedProduct( get_current_user_id(), $product->get_id() ) ) { return false; } return $purchasable; } function hasUserPurchasedProduct( $userId, $productId ) { $orders = wc_get_orders( [ 'customer_id' => $userId, 'status' => [ 'completed', 'processing' ] ] ); foreach ( $orders as $order ) { foreach ( $order->get_items() as $item ) { if ( $item->get_product_id() == $productId ) { return true; } } } return false; }
With this code, customers who have already bought the product won’t be able to add it again.
Block add to cart action and show an error message
If you prefer to block the add-to-cart action and show a message to the user, use the following snippet:
<?php add_filter( 'woocommerce_add_to_cart_validation', 'preventDuplicateProductPurchase', 10, 3 ); function preventDuplicateProductPurchase( $passed, $productId, $quantity ) { if ( is_user_logged_in() && hasUserPurchasedProduct( get_current_user_id(), $productId ) ) { wc_add_notice( __( 'You have already purchased this product. You cannot buy it again.' ), 'error' ); return false; } return $passed; }
This code prevents the product from being added to the cart and displays a notice.
Display a notice above the product page
You can also show a message on the product page to inform the user they’ve already bought the item:
<?php add_action( 'woocommerce_before_single_product', 'showNoticeIfProductPurchased' ); function showNoticeIfProductPurchased() { global $product; if ( is_user_logged_in() && hasUserPurchasedProduct( get_current_user_id(), $product->get_id() ) ) { echo '<div class="woocommerce-message">You have already purchased this product. You cannot buy it again.</div>'; } }
This will show a notice at the top of the product page if the user has already purchased it.
With these small code snippets, you can significantly improve your store’s logic and prevent duplicate purchases—especially useful if you’re selling exclusive or licensed products.