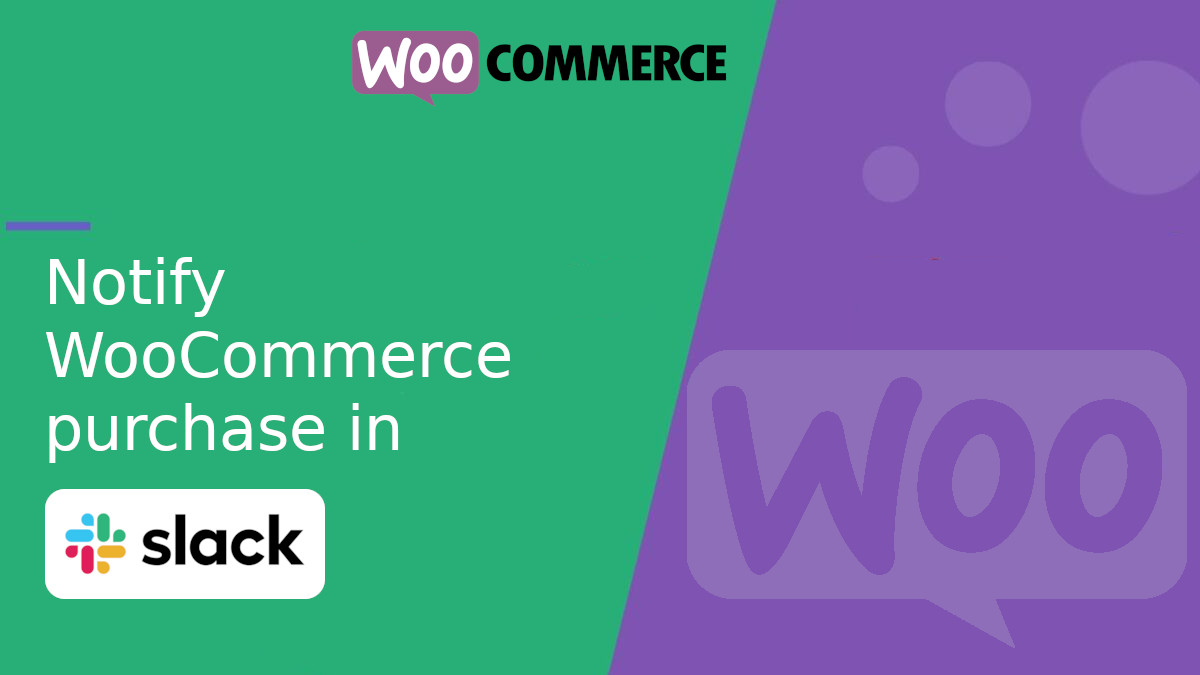
How to Integrate WooCommerce with Slack to receive purchase notifications
If you want to receive Slack notifications whenever a purchase is made in your WooCommerce store, you can easily achieve this using the woocommerce_payment_complete
hook. In this article, I will show you how to integrate WooCommerce with Slack so you receive alerts in a specific channel when a payment is completed.
Prerequisites
- A functioning WooCommerce store.
- A Slack workspace.
- A Slack authentication token to use the modern API.
- The Slack channel ID where messages will be sent.
Create an API Token in Slack
To send messages to Slack, we need to generate an access token:
- Go to Slack API and create a new application.
- In the OAuth & Permissions settings, add the
chat:write
permission (this allows usingchat.postMessage
). - Authorize the application in your workspace.
- Copy the generated authentication token; we will need it in the code.
Get the Slack channel ID
To send messages to a Slack channel, you need its ID. Follow these steps to obtain it:
- Open Slack in the browser or desktop application.
- Go to the channel where you want to receive notifications.
- Click on the channel name at the top.
- Scroll down to the Channel Info section and copy the Channel ID (it will look something like
C0123456789
).
Add the code to WooCommerce
Add the following code to your theme’s functions.php
file or a custom plugin:
<?php function send_slack_notification( $order_id ) { $slack_token = 'xoxb-YOUR-ACCESS-TOKEN'; // Replace with your Slack token $channel_id = 'C0123456789'; // Replace with your Slack channel ID $order = wc_get_order( $order_id ); $message = "📢 *New Purchase Made*\n"; $message .= "🛒 Order #$order_id\n"; $message .= "👤 Customer: " . $order->get_billing_first_name() . " " . $order->get_billing_last_name() . "\n"; $message .= "💰 Total: " . wc_price( $order->get_total() ) . "\n"; $payload = json_encode([ "channel" => $channel_id, "text" => $message ]); $args = [ 'body' => $payload, 'headers' => [ 'Content-Type' => 'application/json', 'Authorization' => 'Bearer ' . $slack_token ], 'timeout' => 10 ]; wp_remote_post( 'https://slack.com/api/chat.postMessage', $args ); } add_action( 'woocommerce_payment_complete', 'send_slack_notification' );
Code explanation
- We get the authentication token generated in Slack.
- We get the channel ID where notifications will be sent.
- We retrieve the order using
wc_get_order( $order_id )
. - We construct the message with the purchase details.
- We send the notification to Slack using the modern
chat.postMessage
API. - We connect the function to the
woocommerce_payment_complete
hook.
Test the integration
- Make a test purchase in your WooCommerce store.
- Verify that the message arrives correctly in the configured Slack channel.
- If you don’t see the notification, check the WordPress error log using
error_log()
.
This integration is an effective way to receive real-time alerts about new sales in your WooCommerce store. If you want to further customize the notification, you can include additional information such as purchased products or payment method.
Try this solution and stay informed about your store’s sales without leaving Slack!