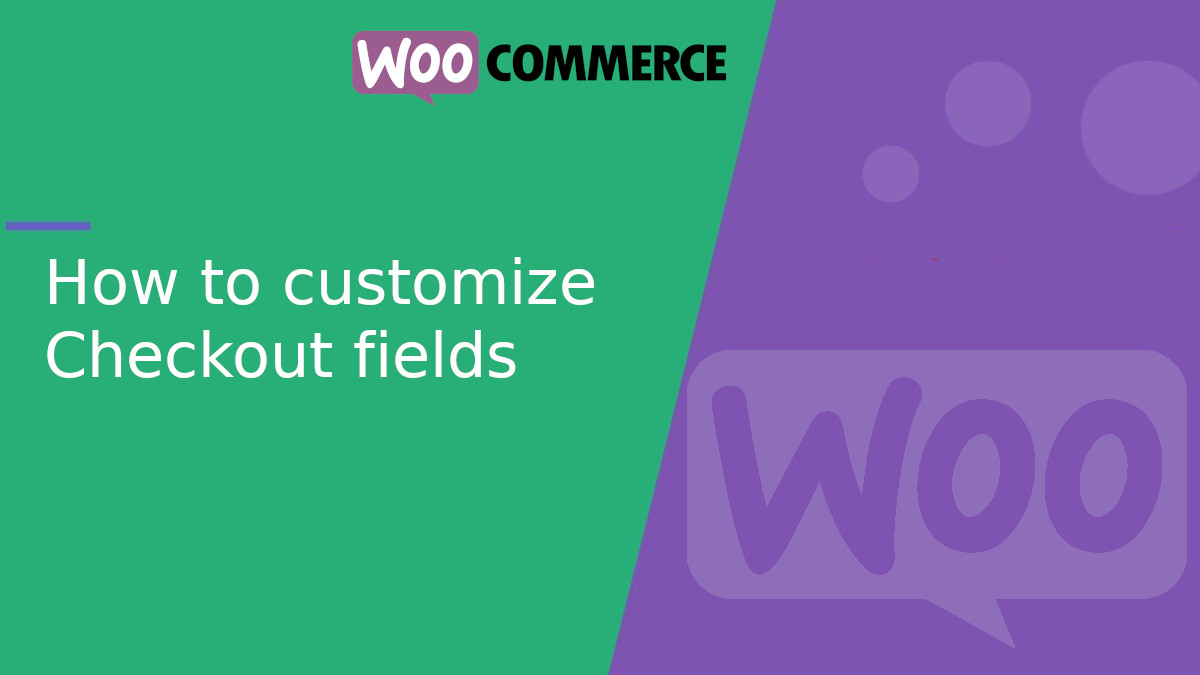
How to customize Checkout fields in WooCommerce
Customizing the checkout page in WooCommerce is essential to improving user experience. WooCommerce allows you to add, remove, and reorder checkout fields using filters and actions.
Adding custom fields to Checkout
We will add two new fields:
billing_type_dni
: A radio button field with “DNI” and “NIT” options.billing_num_dni
: A text field for the identification number.
<?php add_filter( 'woocommerce_checkout_fields', function( $fields ) { // Document type field (DNI or NIT) $fields['billing']['billing_type_dni'] = [ 'type' => 'radio', 'label' => __( 'Document Type', 'woocommerce' ), 'options' => [ 'DNI' => __( 'DNI', 'woocommerce' ), 'NIT' => __( 'NIT', 'woocommerce' ) ], 'required' => true, 'class' => [ 'form-row-wide' ], 'priority' => 20 ]; // Document number field $fields['billing']['billing_num_dni'] = [ 'type' => 'text', 'label' => __( 'Document Number', 'woocommerce' ), 'placeholder' => __( 'Enter your document number', 'woocommerce' ), 'required' => true, 'class' => [ 'form-row-wide' ], 'priority' => 25 ]; return $fields; } );
Removing a field from Checkout
If you don’t want to display a field on the checkout page, you can remove it using unset()
. In this case, we will remove the second address line from the billing section:
<?php add_filter( 'woocommerce_checkout_fields', function( $fields ) { unset( $fields['billing']['billing_address_2'] ); return $fields; } );
Changing the position of a field
WooCommerce orders fields using the priority
property. The lower the number, the higher the field appears in the form.
For example, to display the “Phone” field before “Email”:
<?php add_filter( 'woocommerce_checkout_fields', function( $fields ) { $fields['billing']['billing_phone']['priority'] = 10; $fields['billing']['billing_email']['priority'] = 20; return $fields; } );
Saving custom fields in the order
To ensure that the values entered in billing_type_dni
and billing_num_dni
are saved in the order, we use woocommerce_checkout_update_order_meta
:
<?php add_action( 'woocommerce_checkout_update_order_meta', function( $orderId, $data ) { $order = wc_get_order( $orderId ); if ( ! empty( $data['billing_type_dni'] ) ) { $order->update_meta_data( '_billing_type_dni', sanitize_text_field( $data['billing_type_dni'] ) ); } if ( ! empty( $data['billing_num_dni'] ) ) { $order->update_meta_data( '_billing_num_dni', sanitize_text_field( $data['billing_num_dni'] ) ); } $order->save_meta_data(); }, 10, 2 );
Displaying custom fields in WooCommerce admin
To ensure that the admin can see these fields in the order details, we add them to the billing section:
<?php add_action( 'woocommerce_admin_order_data_after_billing_address', function( $order ) { $typeDni = $order->get_meta( '_billing_type_dni' ); $numDni = $order->get_meta( '_billing_num_dni' ); if ( $typeDni ) { echo '<p><strong>' . __( 'Document Type:', 'woocommerce' ) . '</strong> ' . esc_html( $typeDni ) . '</p>'; } if ( $numDni ) { echo '<p><strong>' . __( 'Document Number:', 'woocommerce' ) . '</strong> ' . esc_html( $numDni ) . '</p>'; } } );
Using these methods, you can easily add custom fields to the WooCommerce checkout page, remove unnecessary fields, and reorder them as needed. You can also ensure that the data is correctly saved and displayed in the WooCommerce admin panel.
Need more examples or have any questions? Feel free to ask!