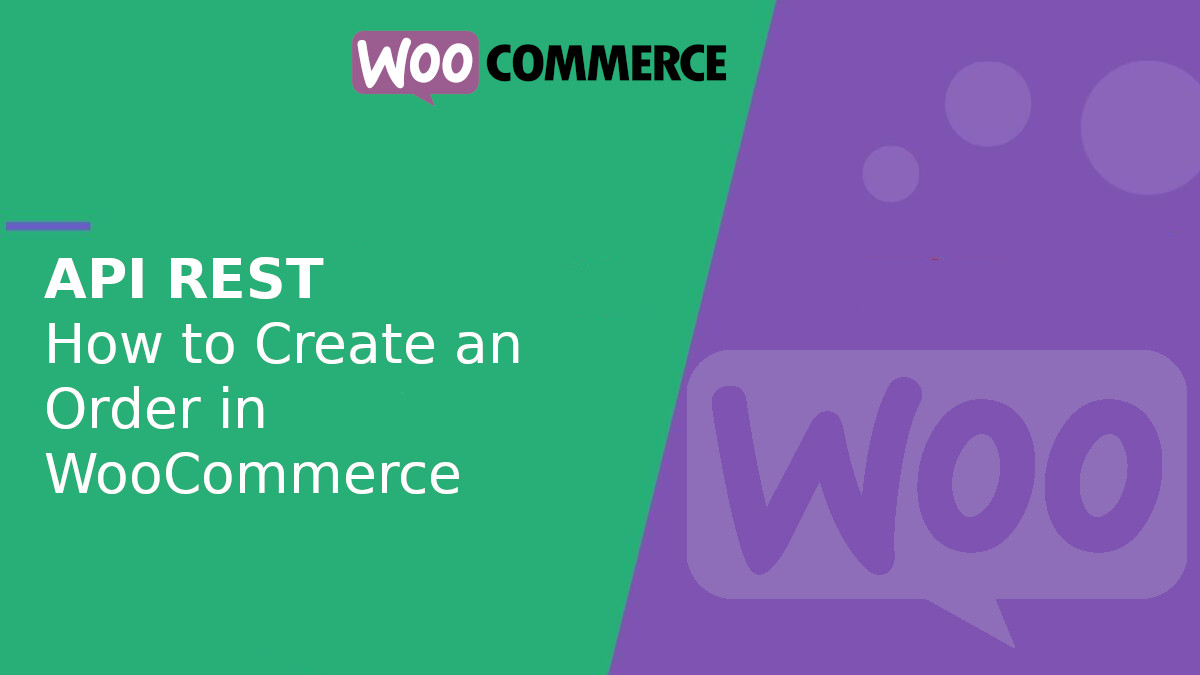
How to create an order in WooCommerce via REST API
WooCommerce provides a powerful REST API that allows you to programmatically interact with your store. In this article, you’ll learn how to create an order in WooCommerce using the REST API, how to add custom meta values, understand the full order response, and what permissions you need.
Creating an order: payload example
Send a POST
request to the /wp-json/wc/v3/orders
endpoint with a payload like this:
{ "payment_method": "bacs", "payment_method_title": "Direct Bank Transfer", "set_paid": false, "billing": { "first_name": "John", "last_name": "Doe", "address_1": "123 Main Street", "city": "New York", "postcode": "10001", "country": "US", "email": "john.doe@example.com", "phone": "123456789" }, "shipping": { "first_name": "John", "last_name": "Doe", "address_1": "123 Main Street", "city": "New York", "postcode": "10001", "country": "US" }, "line_items": [ { "product_id": 123, "quantity": 2 } ], "meta_data": [ { "key": "order_source", "value": "API" } ] }
Tools for consuming the API
Before showing the cURL
example, it’s worth noting that there are many tools you can use to interact with the WooCommerce REST API:
- Postman: Perfect for quick and detailed tests. It provides an easy-to-use interface for building HTTP requests.
- cURL: A popular command-line tool for working with REST APIs.
- Insomnia: Similar to Postman, but with a more minimalist interface. Ideal for developers who prefer lightweight configurations. Download it at Insomnia.
- HTTP Libraries in Programming Languages:
- Axios or Fetch for JavaScript.
- Requests for Python.
- Guzzle for PHP.
These tools make consuming REST APIs efficient, depending on your preferences and needs.
Practical example: creating an order with cURL
If you prefer using the terminal, here’s an example of how to create an order using cURL
:
curl -X POST https://example.com/wp-json/wc/v3/orders \ -u ck_consumer_key:cs_consumer_secret \ -H "Content-Type: application/json" \ -d '{ "payment_method": "bacs", "payment_method_title": "Direct Bank Transfer", "set_paid": false, "billing": { "first_name": "John", "last_name": "Doe", "address_1": "123 Main Street", "city": "New York", "postcode": "10001", "country": "US", "email": "john.doe@example.com", "phone": "123456789" }, "shipping": { "first_name": "John", "last_name": "Doe", "address_1": "123 Main Street", "city": "New York", "postcode": "10001", "country": "US" }, "line_items": [ { "product_id": 123, "quantity": 2 } ], "meta_data": [ { "key": "order_source", "value": "API" } ] }'
Order Response
WooCommerce returns a JSON response with detailed information about the order. Example:
{ "id": 789, "customer_id": 567, "status": "pending", "order_key": "wc_order_abcdef123456", "date_created": "2024-11-29T14:00:00", "date_created_gmt": "2024-11-29T14:00:00", "currency": "USD", "payment_url": "https://example.com/checkout/order-pay/789/?key=wc_order_abcdef123456", "needs_processing": true, "needs_payment": true, "billing": { "first_name": "John", "last_name": "Doe", "email": "john.doe@example.com" }, "line_items": [ { "id": 1, "name": "Sample Product", "quantity": 2, "total": "39.98" } ], "meta_data": [ { "id": 1, "key": "order_source", "value": "API" } ] }
JSON File for Postman
Download a pre-built JSON file to import into Postman for quick testing:
Download JSON File for Postman
Whether you prefer graphical tools like Postman or Insomnia, or direct commands with cURL, the WooCommerce REST API is flexible and powerful. Choose the tools that best fit your workflow to integrate and automate your store.