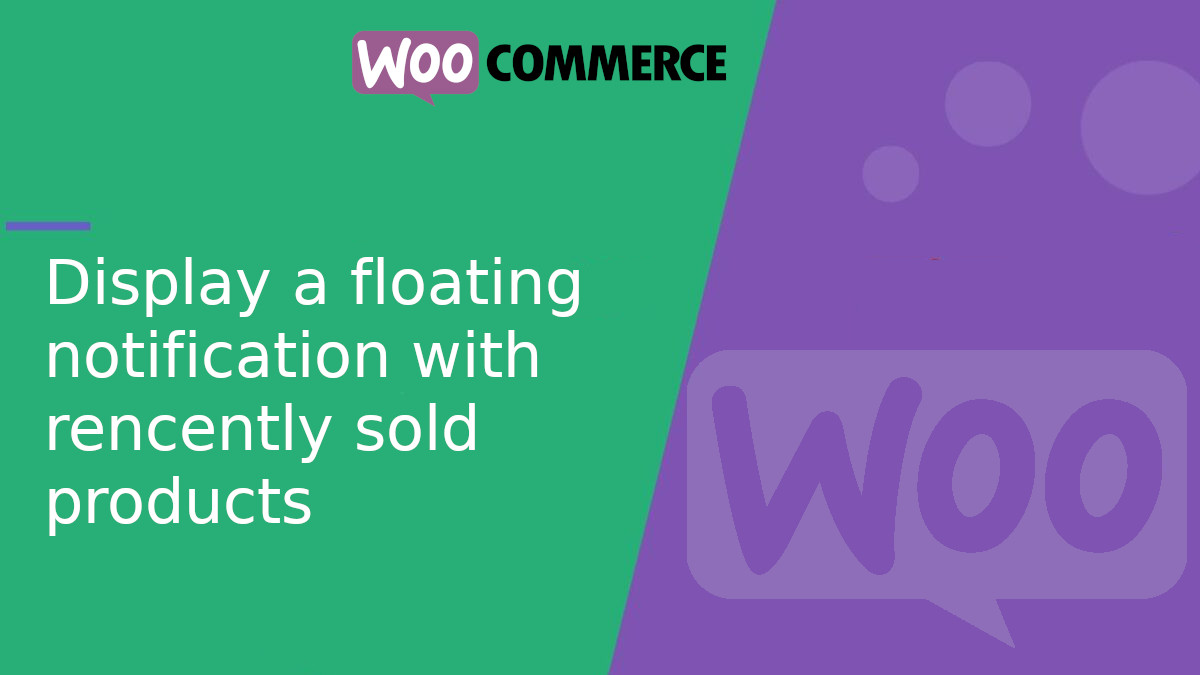
Display a floating notification with recently sold products in WooCommerce
Displaying floating notifications with recently sold products is an excellent way to build trust and create urgency among buyers. In this article, you will learn how to add a floating notification at the bottom of the screen that randomly shows sold products every minute.
How It Works
- A list of the latest sold products in WooCommerce is retrieved.
- A random product from the list is selected.
- A floating notification displaying the product information is shown.
- The notification updates automatically every minute.
Using wc_get_orders
To fetch recently sold products, we will use the wc_get_orders()
function, which allows us to retrieve WooCommerce orders filtered by different criteria. In this case, we will search for completed or processing orders and extract the products sold within them.
PHP Code to Display the Floating Notification
Add the following code to your theme’s functions.php
file or a custom plugin:
<?php function enqueueFloatingNotificationScripts(): void { if ( is_admin() ) { return; } wp_enqueue_style( 'floating-notification-style', get_stylesheet_directory_uri() . '/floating-notification.css' ); wp_enqueue_script( 'floating-notification-script', get_stylesheet_directory_uri() . '/floating-notification.js', ['jquery'], null, true ); wp_localize_script( 'floating-notification-script', 'floatingNotification', [ 'ajaxUrl' => admin_url( 'admin-ajax.php' ), ] ); } add_action( 'wp_enqueue_scripts', 'enqueueFloatingNotificationScripts' ); function getRandomSoldProduct(): void { $args = [ 'limit' => 10, 'orderby' => 'date', 'order' => 'DESC', 'status' => ['wc-completed', 'wc-processing'], ]; $orders = wc_get_orders( $args ); $products = []; foreach ( $orders as $order ) { foreach ( $order->get_items() as $item ) { $productId = $item->get_product_id(); $product = wc_get_product( $productId ); if ( ! $product ) { continue; } $products[] = [ 'id' => $productId, 'name' => $product->get_name(), 'url' => get_permalink( $productId ), 'image' => get_the_post_thumbnail_url( $productId, 'thumbnail' ) ?: wc_placeholder_img_src(), ]; } } if ( empty( $products ) ) { wp_send_json_error(); } $randomProduct = $products[array_rand( $products )]; wp_send_json_success( $randomProduct ); } add_action( 'wp_ajax_get_random_sold_product', 'getRandomSoldProduct' ); add_action( 'wp_ajax_nopriv_get_random_sold_product', 'getRandomSoldProduct' );
CSS for the Floating Notification
Create a file named floating-notification.css
in your theme folder and add:
#floating-notification { position: fixed; bottom: 20px; left: 20px; background: rgba(0, 0, 0, 0.8); color: #fff; padding: 10px; border-radius: 5px; display: none; max-width: 250px; font-size: 14px; z-index: 9999; } #floating-notification img { max-width: 50px; margin-right: 10px; }
JavaScript to Display the Notification Every Minute
Create a file named floating-notification.js
in your theme folder and add:
jQuery(document).ready(function ($) { function fetchProduct() { $.ajax({ url: floatingNotification.ajaxUrl, type: 'POST', data: { action: 'get_random_sold_product' }, success: function (response) { if (response.success) { let product = response.data; let notification = $('#floating-notification'); if (notification.length === 0) { $('body').append('<div id="floating-notification"></div>'); notification = $('#floating-notification'); } notification.html( '<a href="' + product.url + '" style="color:#fff; text-decoration:none;">' + '<img src="' + product.image + '" alt="' + product.name + '"> ' + 'Recently sold: <strong>' + product.name + '</strong></a>' ).fadeIn(400); setTimeout(function () { notification.fadeOut(400); }, 5000); } } }); } fetchProduct(); setInterval(fetchProduct, 60000); });
With this code, your WooCommerce store will display a floating notification showing randomly sold products every minute. You can customize the style and duration according to your needs.