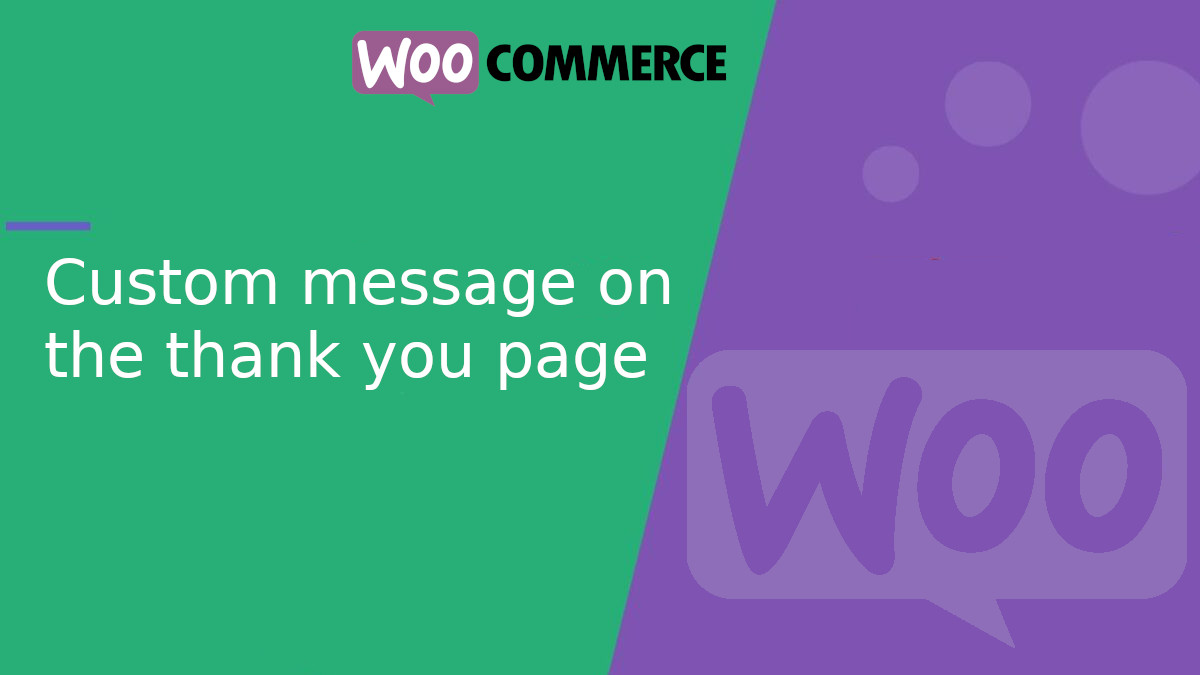
Customize message on the WooCommerce thank you page
The WooCommerce thank you page is the final step of the purchase process, where you can express gratitude to your customers and provide additional relevant information. Customizing this page with a unique message can enhance the user experience and strengthen your relationship with your customers. In this article, we’ll explain how to add a custom message using PHP.
Use the woocommerce_thankyou hook
WooCommerce provides the woocommerce_thankyou
hook, which runs on the thank you page. You can use it to insert additional content, such as a custom message.
Here’s a basic example:
<?php add_action('woocommerce_thankyou', 'add_custom_message_to_thankyou_page'); function add_custom_message_to_thankyou_page($order_id) { // Ensure the order ID is valid. if (!$order_id) { return; } // Retrieve the order details. $order = wc_get_order($order_id); // Ensure the order exists. if ($order) { echo '<p style="font-size: 18px; color: #333;">'; echo __('Thank you for your purchase! Your order is being processed and will be shipped soon.', 'your-text-domain'); echo '</p>'; } }
With this code, a basic thank-you message will appear on the thank you page.
Customize the message based on conditions
You can personalize the message based on order conditions, such as the shipping method, purchased products, or the customer’s country.
Example: Custom Message Based on the Shipping Method
<?php add_action('woocommerce_thankyou', 'add_shipping_based_message_to_thankyou_page'); function add_shipping_based_message_to_thankyou_page($order_id) { if (!$order_id) { return; } $order = wc_get_order($order_id); if ($order) { $shipping_method = $order->get_shipping_method(); if ($shipping_method === 'Free Shipping') { echo '<p style="font-size: 18px; color: green;">'; echo __('Thank you for choosing free shipping! Your order will arrive in 5-7 business days.', 'your-text-domain'); echo '</p>'; } else { echo '<p style="font-size: 18px; color: blue;">'; echo __('Thank you for your order! It will be delivered soon.', 'your-text-domain'); echo '</p>'; } } }
In this example, the message varies depending on whether the customer selected “Free Shipping” or another shipping method.
Display custom information based on purchased products
If you want to show a special message for certain products, you can check if the order contains a specific product.
<?php add_action('woocommerce_thankyou', 'add_product_based_message_to_thankyou_page'); function add_product_based_message_to_thankyou_page($order_id) { if (!$order_id) { return; } $order = wc_get_order($order_id); if ($order) { foreach ($order->get_items() as $item) { $product_id = $item->get_product_id(); if ($product_id == 123) { // Replace 123 with the desired product ID. echo '<p style="font-size: 18px; color: #ff6600;">'; echo __('You purchased our special product! Check your email for exclusive tips.', 'your-text-domain'); echo '</p>'; break; } } } }
This code checks if the order contains a product with a specific ID and displays a special message if it does.
Style the Message
You can style the custom message using HTML and CSS directly in the code. For example:
<?php add_action('woocommerce_thankyou', 'add_styled_message_to_thankyou_page'); function add_styled_message_to_thankyou_page($order_id) { if (!$order_id) { return; } $order = wc_get_order($order_id); if ($order) { echo '<div style="background-color: #f9f9f9; padding: 20px; border: 1px solid #ddd; border-radius: 5px;">'; echo '<h2 style="color: #0056b3;">' . __('Thank You for Your Order!', 'your-text-domain') . '</h2>'; echo '<p style="font-size: 16px; color: #333;">' . __('We appreciate your business. If you have any questions, feel free to contact us.', 'your-text-domain') . '</p>'; echo '</div>'; } }
In this example, the message is wrapped in a styled container to make it visually appealing.
Customizing the WooCommerce thank you page is an excellent way to offer a unique touch to your customers. With the examples above, you can tailor messages to be relevant, visually appealing, and aligned with your store’s needs.
If you need more help or want to explore additional customization options, feel free to ask! 😊