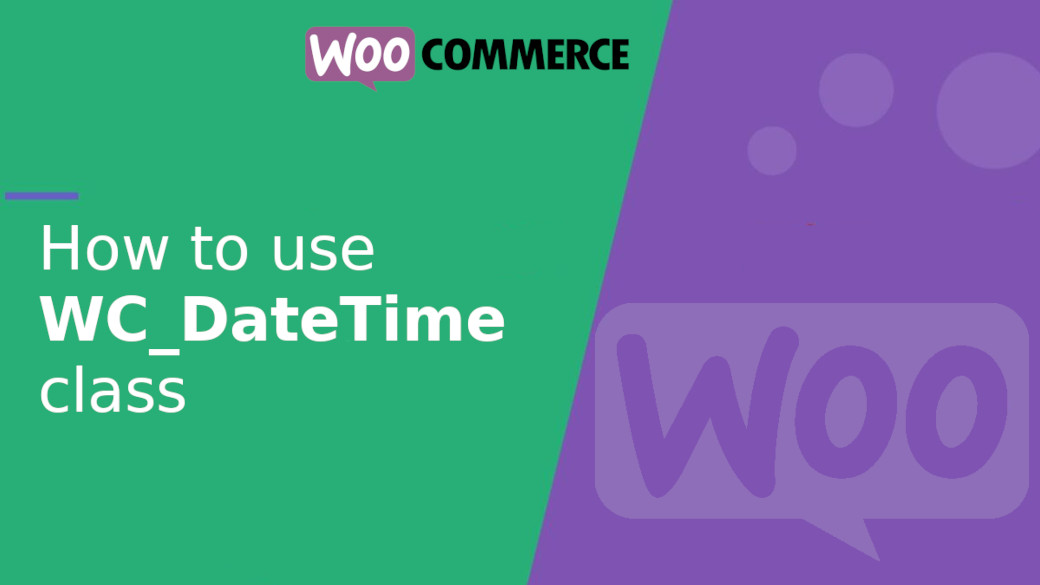
How to use the WC_DateTime class in WooCommerce
The WC_DateTime
class in WooCommerce is a powerful tool for handling dates and times in WooCommerce-related projects. Based on PHP’s native DateTime
class, it includes additional functionalities tailored specifically for the WooCommerce environment.
In this article, we’ll explore how to initialize the class, perform conditional checks, and format date outputs with practical examples.
What is the WC_DateTime Class?
WC_DateTime
extends PHP’s native DateTime
class, adding features such as:
- Compatibility with WooCommerce-specific time zones.
- Simplified methods for formatting dates.
- Seamless handling of conversions between UTC and WordPress-configured time zones.
Initializing a WC_DateTime Object
To create an instance of WC_DateTime
, you can use various initialization methods. Here’s an example:
<?php // Create a WC_DateTime object with the current date $date = new WC_DateTime(); // Create a WC_DateTime object with a specific date $specificDate = new WC_DateTime('2024-11-29 15:30:00'); // Create a WC_DateTime object with a specific time zone $timeZone = new DateTimeZone('America/Lima'); $customDate = new WC_DateTime('2024-11-29 15:30:00', $timeZone); echo $date->format('Y-m-d H:i:s'); // Standard format
Using Conditionals with WC_DateTime
You can compare dates using standard operators ( <
, >
, ==
) or native comparison methods.
Example: Comparing Dates
<?php $today = new WC_DateTime(); $eventDate = new WC_DateTime('2024-12-01 00:00:00'); // Conditional: Check if a date has passed if ($eventDate < $today) { echo "The event has already passed."; } elseif ($eventDate == $today) { echo "The event is today."; } else { echo "The event is in the future."; }
Printing and Formatting Dates with WC_DateTime
The WC_DateTime
class inherits PHP’s format()
method for customizing outputs. It also offers additional methods like getTimestamp()
.
Example: Formatting Dates
<?php $date = new WC_DateTime('2024-11-29 15:30:00'); // Custom format echo $date->format('d/m/Y H:i'); // Output: 29/11/2024 15:30 // Get UNIX timestamp echo $date->getTimestamp(); // Output: 1732889400 // Convert to WordPress time zone $date->setTimezone(wc_timezone_string()); echo $date->format('Y-m-d H:i:s'); // Adjusted format
Common Use Cases for WC_DateTime in WooCommerce
This class is useful for handling dates in various WooCommerce-related functionalities. Here are some practical use cases:
Example: Get the Creation Date of an Order
<?php $order = wc_get_order(123); // Replace 123 with the order ID $orderDate = $order->get_date_created(); // Returns a WC_DateTime object echo $orderDate->format('d/m/Y H:i'); // Output: Order creation date
Example: Check if a Subscription Has Expired
<?php $subscription = wcs_get_subscription(456); // Replace 456 with the subscription ID $endDate = $subscription->get_date('end'); // Get the end date if ($endDate && new WC_DateTime() > $endDate) { echo "The subscription has expired."; } else { echo "The subscription is active."; }
The WC_DateTime
class is a flexible and essential tool for handling dates and times in WooCommerce. Its integration with WordPress and WooCommerce configurations simplifies tasks like date comparisons, formatting, and managing time zones.
If you’re developing custom functionalities for WooCommerce, such as plugins or themes, mastering WC_DateTime
will allow you to handle dates efficiently and professionally.
Have questions or feedback? Leave them in the comments below!